Event Listener in Google Charts API
Solution 1
Here is a working version.
There was no listener to the select event, and you mixed up, data
and table
for the getValue
call.
<html>
<head>
<script src='https://www.google.com/jsapi'></script>
<script>
google.load('visualization', '1', {packages:['table']});
google.setOnLoadCallback(drawTable);
var table, data;
function drawTable() {
data = new google.visualization.DataTable();
data.addColumn('string', 'Name');
data.addColumn('number', 'Salary');
data.addColumn('boolean', 'Full Time Employee');
data.addRows(4);
data.setCell(0, 0, 'Mike');
data.setCell(0, 1, 10000, '$10,000');
data.setCell(0, 2, true);
data.setCell(1, 0, 'Jim');
data.setCell(1, 1, 8000, '$8,000');
data.setCell(1, 2, false);
data.setCell(2, 0, 'Alice');
data.setCell(2, 1, 12500, '$12,500');
data.setCell(2, 2, true);
data.setCell(3, 0, 'Bob');
data.setCell(3, 1, 7000, '$7,000');
data.setCell(3, 2, true);
table = new google.visualization.Table(document.getElementById('table_div'));
table.draw(data, {showRowNumber: true});
//add the listener
google.visualization.events.addListener(table, 'select', selectionHandler);
}
function selectionHandler() {
var selectedData = table.getSelection(), row, item;
row = selectedData[0].row;
item = data.getValue(row,0);
alert("You selected :" + item);
}
</script>
</head>
<body>
<div id='table_div'></div>
<input type="button" value="Select" onClick="selectionHandler()">
</body>
</html>
Solution 2
Hard for me to know what wrong with your code, here example code from the documentation explain how to handle select event:
// Create our table.
var table = new google.visualization.Table(document.getElementById('table_div'));
table.draw(data, options);
// Add our selection handler.
google.visualization.events.addListener(table, 'select', selectHandler);
// The selection handler.
// Loop through all items in the selection and concatenate
// a single message from all of them.
function selectHandler() {
var selection = table.getSelection();
var message = '';
for (var i = 0; i < selection.length; i++) {
var item = selection[i];
if (item.row != null && item.column != null) {
var str = data.getFormattedValue(item.row, item.column);
message += '{row:' + item.row + ',column:' + item.column + '} = ' + str + '\n';
} else if (item.row != null) {
var str = data.getFormattedValue(item.row, 0);
message += '{row:' + item.row + ', column:none}; value (col 0) = ' + str + '\n';
} else if (item.column != null) {
var str = data.getFormattedValue(0, item.column);
message += '{row:none, column:' + item.column + '}; value (row 0) = ' + str + '\n';
}
}
if (message == '') {
message = 'nothing';
}
alert('You selected ' + message);
}
You can give it try live code from jsfiddle
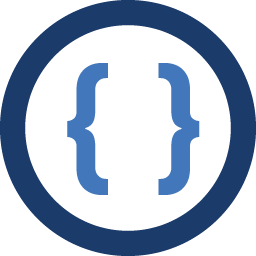
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
I'm busy using Google Charts in one of my projects to display data in a table. Everything is working great. Except that I need to see what line a user selected once they click a button.
This would obviously be done with Javascript, but I've been struggling for days now to no avail. Below I've pasted code for a simple example of the table, and the Javascript function that I want to use (that doesn't work).
<html> <head> <script type='text/javascript' src='https://www.google.com/jsapi'></script> <script type='text/javascript'> google.load('visualization', '1', {packages:['table']}); google.setOnLoadCallback(drawTable); var table = ""; function drawTable() { var data = new google.visualization.DataTable(); data.addColumn('string', 'Name'); data.addColumn('number', 'Salary'); data.addColumn('boolean', 'Full Time Employee'); data.addRows(4); data.setCell(0, 0, 'Mike'); data.setCell(0, 1, 10000, '$10,000'); data.setCell(0, 2, true); data.setCell(1, 0, 'Jim'); data.setCell(1, 1, 8000, '$8,000'); data.setCell(1, 2, false); data.setCell(2, 0, 'Alice'); data.setCell(2, 1, 12500, '$12,500'); data.setCell(2, 2, true); data.setCell(3, 0, 'Bob'); data.setCell(3, 1, 7000, '$7,000'); data.setCell(3, 2, true); table = new google.visualization.Table(document.getElementById('table_div')); table.draw(data, {showRowNumber: true}); } function selectionHandler() { selectedData = table.getSelection(); row = selectedData[0].row; item = table.getValue(row,0); alert("You selected :" + item); } </script> </head> <body> <div id='table_div'></div> <input type="button" value="Select" onClick="selectionHandler()"> </body> </html>
Thanks in advance for anyone taking the time to look at this. I've honestly tried my best with this, hope someone out there can help me out a bit.