how to implement protocol delegate pattern in App Delegate when using Platform Specific code in Flutter?
344
I can solve it by using the code like this by using extension. please scroll to the very bottom to see FlutterViewController extension
@UIApplicationMain
@objc class AppDelegate: FlutterAppDelegate {
override func application(
_ application: UIApplication,
didFinishLaunchingWithOptions launchOptions: [UIApplication.LaunchOptionsKey: Any]?
) -> Bool {
let controller : FlutterViewController = window?.rootViewController as! FlutterViewController
setUpMethodChannels(controller: controller) // set the method channel
GeneratedPluginRegistrant.register(with: self)
return super.application(application, didFinishLaunchingWithOptions: launchOptions)
}
private func setUpMethodChannels(controller: FlutterViewController) {
let channel = FlutterMethodChannel(name: "useOtherApp", binaryMessenger: controller.binaryMessenger)
channel.setMethodCallHandler({ (call: FlutterMethodCall, result: FlutterResult) -> Void in
guard let args = call.arguments as? [String : Any] else {return}
if (call.method == "saveToCalendar") {
let eventVC = EKEventEditViewController()
eventVC.editViewDelegate = controller // set FlutterViewController as the delegate
eventVC.eventStore = EKEventStore()
let event = EKEvent(eventStore: eventVC.eventStore)
eventVC.event = event
eventVC.title = "hallooooo"
controller.present(eventVC, animated: true)
} else {
result(FlutterMethodNotImplemented)
}
})
}
}
// set the extension like below OUTSIDE the AppDelegate class
extension FlutterViewController : EKEventEditViewDelegate, UINavigationControllerDelegate {
// conform to the EKEventEditViewDelegate protocol
public func eventEditViewController(_ controller: EKEventEditViewController, didCompleteWith action: EKEventEditViewAction) {
dismiss(animated: true, completion: nil)
}
}
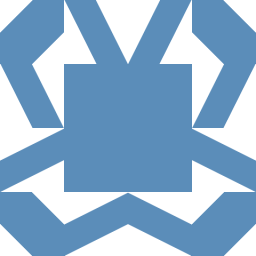
Author by
Alexa289
Updated on December 30, 2022Comments
-
Alexa289 5 months
I want to implement platform specific code for my Flutter app on iOS. I want to implement EventKit from native iOS. but now I am confused how to implement protocol delegate pattern in the app delegate when using Platform Channel in Flutter.
in native iOS, my code will be like this
import EventKitUI class MyViewController : UIViewController, EKEventEditViewDelegate, UINavigationControllerDelegate { override func viewDidLoad() { super.viewDidLoad() } @IBAction func buttonPressed(_ sender: Any) { // after pressing a button then show a VC showEventKitViewController() } func eventEditViewController(_ controller: EKEventEditViewController, didCompleteWith action: EKEventEditViewAction) { // this is the method to conform the protocol dismiss(animated: true, completion: nil) } func showEventKitViewController() { let eventVC = EKEventEditViewController() eventVC.editViewDelegate = self // I am confused in this line eventVC.eventStore = EKEventStore() let event = EKEvent(eventStore: eventVC.eventStore) eventVC.event = event present(eventVC, animated: true) } }
as you can see, I assign
self
( MyViewController class as the delegate ) foreditViewDelegate
now I am confused how to implement
showEventKitViewController
method above in Flutter app delegate below@UIApplicationMain @objc class AppDelegate: FlutterAppDelegate { override func application( _ application: UIApplication, didFinishLaunchingWithOptions launchOptions: [UIApplication.LaunchOptionsKey: Any]? ) -> Bool { let controller : FlutterViewController = window?.rootViewController as! FlutterViewController let channel = FlutterMethodChannel(name: "useOtherApp", binaryMessenger: controller.binaryMessenger) channel.setMethodCallHandler({ (call: FlutterMethodCall, result: FlutterResult) -> Void in if (call.method == "saveToEventKit") { // what should I do in here to get the same result like my code above in native? } }) }
especially when I need to assign a class as the delegate for
editViewDelegate
like this-
sarah almost 2 yearsI have the same question :(
-