How to insert image on UIView?
21,354
Solution 1
If you want to add image on view. Please use below code.
let imageName = "yourImage.png"
let image = UIImage(named: imageName)
let imageView = UIImageView(image: image!)
imageView.frame = CGRect(x: 0, y: 0, width: 100, height: 200)
self.view.addSubview(imageView)
//Imageview on Top of View
self.view.bringSubview(toFront: imageView)
If you want to draw specific shape then go with Core Graphics
https://developer.apple.com/documentation/coregraphics
https://cocoacasts.com/drawing-shapes-in-swift-with-paintcode/
https://www.ioscreator.com/tutorials/drawing-shapes-core-graphics-tutorial-ios10
Solution 2
Add UIImage
on the view layer
let myLayer = CALayer()
let myImage = UIImage(named: "star")?.cgImage
myLayer.frame = CGRect(x: 0, y: 0, width: 60, height: 60)
myLayer.contents = myImage
myView.layer.addSublayer(myLayer)
Add UIImage
as a subView
let image = UIImage(named: "star")
let imageView = UIImageView(image: image!)
imageView.frame = CGRect(x: 0, y: 0, width: 60, height: 60)
myView.addSubview(imageView)
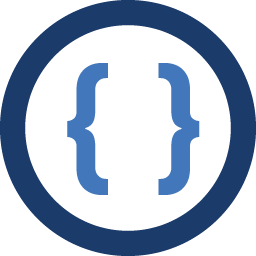
Author by
Admin
Updated on May 18, 2020Comments
-
Admin almost 4 years
The code below prints a line on a UIView. I just want to know the code that I would write to be able to insert an image on top of the view.
import UIKit class draw: UIView { var line = UIBezierPath() var line1 = UIBezierPath() func grapher() { line1.move(to: .init(x:0, y: bounds.height / 6)) line1.addLine(to: .init(x: bounds.width, y: bounds.height / 6)) UIColor.blue.setStroke() line1.lineWidth = 2 line1.stroke() } override func draw(_ rect: CGRect) { grapher() } }