How to invoke flutter method from broadcastReceiver class?
1,127
Basically you can't access methodChannel in BroadcastReceiver directly so you have to make methodChannel in compaion object So,
Add these lines to your MainActivity
companion object {
lateinit var methodChannel: MethodChannel
}
And in onCreate method of MainActivity replace
val methodChannel = MethodChannel(flutterView, channel)
To :
methodChannel = MethodChannel(flutterView, channel)
now you can use MainActivity.methodChannel anywhere in your app.
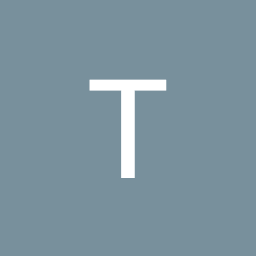
Author by
Titas Černiauskas
Updated on December 24, 2022Comments
-
Titas Černiauskas over 1 year
I am trying to awake flutter method on a received call. I already done the receiving part, but I can't awake flutter method yet.
I tried calling method channel in onReceive() method in MainActivity.kt class, but it gives me error. method channel only seems to work in onCreate() method.
The question is how can I invoke flutter method in onReceive() or is there another way to do it?
MainActivity.kt
import android.Manifest import android.content.BroadcastReceiver import android.content.Context import android.content.Intent import android.content.IntentFilter import android.content.pm.PackageManager import android.os.Build import android.os.Bundle import android.widget.Toast import androidx.annotation.RequiresApi import androidx.core.app.ActivityCompat import androidx.core.content.ContextCompat import io.flutter.app.FlutterActivity import io.flutter.plugin.common.MethodCall import io.flutter.plugin.common.MethodChannel import io.flutter.plugins.GeneratedPluginRegistrant class MainActivity: FlutterActivity(){ var updateUIReciver: BroadcastReceiver? = null @RequiresApi(Build.VERSION_CODES.O) override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) GeneratedPluginRegistrant.registerWith(this) registerReceiver(broadcastReceiver, IntentFilter("Service.to.activity")); val channel = "my.data" val methodChannel = MethodChannel(flutterView, channel) val map: HashMap<String, String> = HashMap() val permissionCheck: Int = ContextCompat.checkSelfPermission(this, Manifest.permission.READ_PHONE_STATE) if (permissionCheck == PackageManager.PERMISSION_GRANTED) { Toast.makeText(this, "Permission granted ", Toast.LENGTH_LONG).show(); } else { //TODO ActivityCompat.requestPermissions(this, arrayOf(Manifest.permission.READ_PHONE_STATE), 4); Toast.makeText(this, "Permission not granted ", Toast.LENGTH_LONG).show(); } methodChannel.setMethodCallHandler { call: MethodCall, result: MethodChannel.Result? -> if (call.method == "callMyFunction") { methodChannel.invokeMethod("callMyFunction", map) } else { } } } var broadcastReceiver: BroadcastReceiver = object : BroadcastReceiver() { override fun onReceive(context: Context, intent: Intent) { Toast.makeText(context, "Incoming call received", Toast.LENGTH_LONG).show() // I can't call "methodChannel.invokeMethod("callMyFunction", map)" here cause of error. } } }
MyBroadcastReceiver.kt
import android.app.Service import android.content.BroadcastReceiver import android.content.Context import android.content.Intent import android.telephony.PhoneStateListener import android.telephony.TelephonyManager import androidx.core.app.NotificationCompat import android.app.NotificationManager; import android.os.Build; import android.os.IBinder; import android.widget.Toast class MyBroadcastReceiver : BroadcastReceiver() { override fun onReceive(context: Context, intent: Intent) { val telephony = context.getSystemService(Service.TELEPHONY_SERVICE) as TelephonyManager telephony.listen(object : PhoneStateListener() { override fun onCallStateChanged(state: Int, incomingNumber: String) { super.onCallStateChanged(state, incomingNumber) context.sendBroadcast(Intent("Service.to.activity")) } }, PhoneStateListener.LISTEN_CALL_STATE) } }
Flutter code
const platform = const MethodChannel('my.data'); Future<void> _receiveFromNative(MethodCall call) async { try { if (call.method == "callMyFunction") { print("Received in flutter"); } } on PlatformException catch (e) {} } platform.setMethodCallHandler(_receiveFromNative);