How to listen for user generated zoom in Google Maps?
Solution 1
I wouldn't just hook in to the +
/-
buttons (or buttons on your own custom control for that matter). The user can change the zoom on the map with the mouse wheel, or by double-clicking on the map. Plus, you'd be relying on implementation detail rather than documented API, which is a major no-no.
This really means the only reliable, documented way to detect a change in zoom is to listen to the zoom_changed
event of the Map
.
If your event handler can't determine whether the event came from user action or an API call, there's two approaches:
- Set a flag before calling an API function so that you know you can ignore this change.
- Can you re-architect your app such that it does not matter whether a zoom change came from code or the user?
Solution 2
I solved this issue by creating Custom Zoom buttons for my Map.
Here is the code from my project:
Edit: Removed unnecessary and self explanatory common code
your zoomControl function:
function zoomControl(map, div) {
var controlDiv = div,
control = this;
// Set styles to your own pa DIV
controlDiv.style.padding = '5px';
// Set CSS for the zoom in div.
var zoomIncrease = document.createElement('div');
zoomIncrease.title = 'Click to zoom in';
// etc.
// Set CSS for the zoom in interior.
var zoomIncreaseText = document.createElement('div');
// Use custom image
zoomIncreaseText.innerHTML = '<strong><img src="./images/plusBut.png" width="30px" height="30px"/></strong>';
zoomIncrease.appendChild(zoomIncreaseText);
// Set CSS for the zoom out div, in asimilar way
var zoomDecreaseText = document.createElement('div');
// .. Code .. Similar to above
// Set CSS for the zoom out interior.
// .. Code ..
// Setup the click event listener for Zoom Increase:
google.maps.event.addDomListener(zoomIncrease, 'click', function() {
zoom = MainMap.getZoom();
MainMap.setZoom(zoom+1);
// Other Code parts
});
// Setup the click event listener for Zoom decrease:
google.maps.event.addDomListener(zoomDecrease, 'click', function() {
zoom = MainMap.getZoom();
MainMap.setZoom(zoom-1);
});
}
your initialize function:
function initializeMap() {
var latlng = new google.maps.LatLng(38.6, -98);
var options = {
zoom : 5,
center : latlng,
mapTypeId : google.maps.MapTypeId.ROADMAP,
// Other Options
};
MainMap = new google.maps.Map(document.getElementById("google-map-canvas"),
options);
// The main part - Create your own Custom Zoom Buttons
// Create the DIV to hold the control and call the zoomControl()
var zoomControlDiv = document.createElement('div'),
zoomLevelControl = new zoomControl(MainMap, zoomControlDiv);
zoomControlDiv.index = 1;
MainMap.controls[google.maps.ControlPosition.RIGHT_TOP].push(zoomControlDiv);
}
Hope it helps
Solution 3
var zoomFlag = "user"; // always assume it's user unless otherwise
// some method changing the zoom through API
zoomFlag = "api";
map.setZoom(map.getZoom() - 1);
zoomFlag = "user";
// google maps event handler
zoom_changed: function() {
if (zoomFlag === "user") {
// user zoom
}
}
Solution 4
I was looking to do the same thing. I was hoping to find that there was a way built into the Google Maps API, but at a minimum, you should be able to store the starting zoom level as a variable when you initialize the map. Then, compare the result of getZoom() to it to know whether it was a zoom in or a zoom out.
For example:
map = new google.maps.Map(document.getElementById('map_canvas'), { zoom: 11 });
var previous_zoom = 11;
google.maps.event.addListener(map,'zoom_changed',function(){
if(map.getZoom() > previous_zoom) {
alert('You just zoomed in.');
}
previous_zoom = map.getZoom();
}
Related videos on Youtube
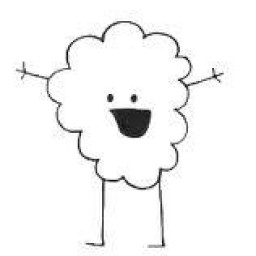
LAW
Updated on June 05, 2022Comments
-
LAW about 1 year
I want to know when a Google Maps zoom_changed event is fired specifically by a user interaction with the +/- zoom buttons. If I use a general event listener for zoom_changed, I can't tell if it is a user-generated event or a zoom change caused by something like fitBounds(). Looking for the best way to do this.
I've tried the following things, none of which seem to work:
1) Looked for event information on zoom_changed. There appears to be none.
2) Add listeners for mouseover and mouseout that let me set a flag to see if the user is in the map bounds, and check the flag on zoom_changed. This doesn't work because the map does not consider the zoom buttons as part of the map frame (in other words, hovering over zoom buttons triggers the mouseout event).
3) Add a normal (non-gMap) listener to the zoom buttons. However, I can't find a definitive CSS selector that will allow me to grab just the buttons.
4) Looked for a function in the gMaps API that would let me do something like getZoomElements(), and then I could set listeners using that.
The weird thing is I can clearly do what I want if I add a custom control to the map. It seems very odd that they would force me to do that instead of having a hook into the default zoom controls.
-
josh3736 about 11 yearsAlso consider the mouse wheel -- there's more than one way for a user to manipulate the zoom.
-
-
josh3736 about 11 yearsThis doesn't work because button controls (whether built-in or custom) aren't the only way a user can change the zoom on a map, as I outlined.
-
andresf about 11 yearsPerhaps you and I are interpreting the original poster's question differently. The first line of his question: "I want to know when a Google Maps zoom_changed event is fired specifically by a user interaction with the +/- zoom buttons." He only wants to know when the user clicked on the controls...not via mouse wheel or other. Salient part of his question: "specifically by a user interaction with the +/- buttons."
-
josh3736 about 11 yearsRead the second sentence. What he's really trying to do is differentiate between
zoom_changed
caused by user interaction (whatever the source) and API calls. -
andresf about 11 yearsI'm afraid it doesn't sound that way to me. So the second sentence states that he's tried to find out if a user clicked on the control through
zoom_changed
, but for obvious reasons that returns an ambiguous result. Anyway, I think at this point it's worth leaving it up to the OP to determine whether we've interpreted his question and intent properly. -
Kush almost 9 yearsthis may not be helpful. I have a similar case where I preset zoom level to 12 and on zoom_changed event I compare the new and prev zoom values. When fitBounds (even when user did not interacted with map for example: when map is trying to fit markers while loading) is called the zoom level might change and this condition will still be successful and it seems like user wanted to zoom in/out which is not true.