How to make a dynamic bootstrap pagination with JS?
Solution 1
I suggest you use bootpag,
It's very simple and works good with bootstrap, it also has good documentation to show you how to use it step by step.
I don't have to explain it here since everything is explained on the website.
I hope this can help you.
Link: http://botmonster.com/jquery-bootpag/#pro-page-8
Solution 2
Html
<table class="table">
<thead>
<tr>
<th scope="col">#</th>
<th scope="col">Name</th>
<th scope="col">Salary</th>
<th scope="col">Age</th>
</tr>
</thead>
<tbody class="page-data">
</tbody>
</table>
<nav aria-label="Page navigation example">
<ul class="pagination">
<li onclick="prePage()" class="page-item page-list">
<a class="page-link" href="#" aria-label="Previous">
<span aria-hidden="true">«</span>
<span class="sr-only">Previous</span>
</a>
</li>
<li onclick="nextPage()" class="page-item">
<a class="page-link" href="#" aria-label="Next">
<span aria-hidden="true">»</span>
<span class="sr-only">Next</span>
</a>
</li>
</ul>
</nav>
JS Code
<script>
let userData = null;
$.ajax({
url: "http://dummy.restapiexample.com/api/v1/employees",
type: "get",
async: false,
success: function(users) {
userData = users.data;
}
});
var currentPage = 0;
let pages = "";
let page_size = 5;
pages = paginate(userData, page_size);
pageLi = "";
pages.forEach((element, index) => {
if (index != 0)
pageLi += '<li onclick="pageChange(' + index + ')" id="page_' + index + '" class="page-item list-item" id="page_' + index + '"><a class="page-link" href="javascript:void(0)">' + index + '</a></li>';
});
$(".page-list").after(pageLi);
page = pages[currentPage];
printRows(page);
function nextPage() {
if (pages.length - 1 > currentPage)
page = currentPage + 1;
pageChange(page);
}
function prePage() {
if (currentPage < pages.length && currentPage != 0)
page = currentPage - 1;
pageChange(page);
}
function pageChange(page) {
currentPage = page;
$(".list-item").removeClass("active");
$("#page_" + page).addClass("active");
$(".page-data").html("");
page = pages[page];
printRows(page);
}
function printRows(arr) {
arr.forEach(element => {
$(".page-data").append("<tr><td>" + element.id + "</td><td>" + element.employee_name + "</td><td>" + element.employee_salary + "</td><td>" + element.employee_age + "</td></tr>");
});
}
function paginate(arr, size) {
return arr.reduce((acc, val, i) => {
let idx = Math.floor(i / size)
let page = acc[idx] || (acc[idx] = [])
page.push(val)
return acc
}, [])
}
</script>
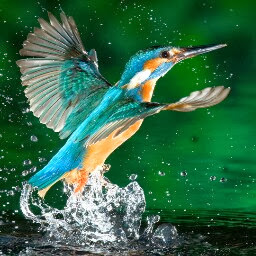
Demirro
Updated on June 04, 2022Comments
-
Demirro almost 2 years
So basically I call on this 840 Json Object dataset with AJAX and display it using divs and a simple enough bootstrap pagination. Now my issue is, that I have 94 pages and all the page buttons are getting displayed all the time. I think that is neither practical, pretty or user-friendly so I'd like to fix that.
So I've scoured the internet about this issue. I've found several pagination plugins supposed to deliver exactly what I need like simplePagination.js or twbsPagination. The latter worked the best for me but still didn't function correctly. I was able to get the new pagination up and running but it would not change the actual page content. I have now tried many more plugins and tried modifying my existing code but nothing worked. I reverted my code back to the normal pagination now.
pageSize = 9; var pageCount = $(".line-content").length / pageSize //calculating the number of pages needed var pagin = document.getElementById('pagin'); //deleting the previous displayed page buttons while (pagin.firstChild) { pagin.removeChild(pagin.firstChild); } for (var i = 0; i < pageCount; i++) { //creating the page items $("#pagin").append('<li class="page-item"><a class="page-link" href="#">' + (i + 1) + '</a></li> '); } //styling the current page item $("#pagin li").first().find("a").addClass("current") // the function to actually change the content on the selected page showPage = function (page) { $(".line-content").hide(); $(".line-content").each(function (n) { if (n >= pageSize * (page - 1) && n < pageSize * page) $(this).show(); }); } showPage(1); //displaying the content //changing the style $("#pagin li a").click(function () { $("#pagin li a").removeClass("current"); $(this).addClass("current"); showPage(parseInt($(this).text())) });
So basically I got this working pagination but I'd like to get it sleeker. I'm open to any jquery plugins and everything. As long as it does what I hope it can. My pagination looks like this I'd like to have it looking somewhat like this
-
Shujaat Ali over 2 yearsGood one! helped me a lot creating custom component with vue js 1.