Using ajax to send form data to php
10,535
Solution 1
You can use serialize method on form, it will gather everything correct.
$('#signup').live('click', function(){
var that = $('form.check-user'),
urls = that.attr('action'),
methods = that.attr('method'),
data = that.serialize();
$.ajax(
{
url: urls,
type: methods,
data : data,
beforeSend: function(response){alert('Sending');},
success: function(response){ alert('success');},
error: function(response){alert('failed');},
complete: function(response){alert('finished');},
}
);
return false;
});
Solution 2
Try this,
$('#signup').live('click', function(){
$.ajax({
url:’’,//url to submit
type: "post",
dataType : 'json',
data : {
'Susername' : $('#Susername').val(),
'Semail' : $('#Semail').val(),
'Spassword' : $('#Spassword').val()
},
success: function (data)
{
}
});
return false;
});
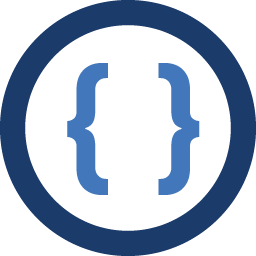
Author by
Admin
Updated on June 15, 2022Comments
-
Admin almost 2 years
I want to send form data from ajax to php. My ajax retrieves the form data but it doesnt send it i dont see anything wrong with the code maybe i need a much more professional help. Thanks in advance
HTML5 syntax
<div align="center"><a id="hi">Header</a></div> <a id="signup" data-add-back-btn="true" style="float:right;" data-icon="arrow-r">Sign- In</a> </div> <form class="check-user" action="php/sup.php" method="POST"> <label>Username</label> <input id="Susername" name="username" placeholder="username" type="text" > </div> <div align="center" data-role="fieldcontain" style="width:100%;overflow:hidden" data-position="static"> <label>Email</label> <input id="Semail" name="email" placeholder="email" type="email" > </div> <div align="center" data-role="fieldcontain" style="width:100%;overflow:hidden" data-position="static"> <label>Password</label> <input id="Spassword" name="password" placeholder="password" type="password" > </div> <!---input type="submit" style="visibility:hidden;" id="send"/--> </form>
Ajax syntax
$('#signup').live('click', function(){ //var name = document.getElementById('Susername').value; //var email = document.getElementById('Semail').value; //var pass = document.getElementById('Spassword').value; var that = $('form.check-user'), urls = that.attr('action'), methods = that.attr('method'), data = {}; that.find('[name]').each(function(index, element) { var that = $(this), name = that.attr('name'), element = that.val(); alert(name+'='+element+' '+methods); data[name] = element; }); $.ajax( { url: urls, type: methods, data : data, beforeSend: function(response){alert('Sending');}, success: function(response){ alert('success');}, error: function(response){alert('failed');}, complete: function(response){alert('finished');}, } ); return false; });
PHP syntax
session_start(); $name = $_POST['username']; $email = $_POST['email']; $password = $_POST['password']; if(isset($name) && isset($email) && isset($password)) { echo $name; $_SESSION['username'] = $name; } else { die('data not set'); }