How to make a dynamic dropdownbutton list in Flutter ? (delete items)
Use a setState()
method, so every time this method is called, your DropDownButton
is redrawn.
Here is the Solution you're looking for :
- I've demonstrated it with an
ElevatedButton()
which on click updates your list values with your "Firestore values" and redraws the DropDownButton thanks tosetState()
method :
import 'package:flutter/cupertino.dart';
import 'package:flutter/material.dart';
class DropdownMenu extends StatefulWidget {
const DropdownMenu({Key? key}) : super(key: key);
@override
State<DropdownMenu> createState() => _DropdownMenuState();
}
/// This is the private State class that goes with MyStatefulWidget.
class _DropdownMenuState extends State<DropdownMenu> {
var dropdownValue;
String hintValue = 'Tout de Suite';
List<String> values = [
'Initial value 1',
"Initial Value 2",
'Initial value 3',
"Initial Value 4",
];
@override
Widget build(BuildContext context) {
return Column(
children: [
ElevatedButton(
onPressed: () {
setState(() {
values = [
'New value from Firebase',
"Values edited from firebase"
];
});
},
child: Text("Update with current Firestore values "),
),
DropdownButton<String>(
value: dropdownValue,
hint: Text(
hintValue,
),
underline: Container(
height: 0,
),
onChanged: (String? newValue) {
setState(() {
hintValue = newValue!;
});
},
items: values.map<DropdownMenuItem<String>>((String value) {
return DropdownMenuItem<String>(
value: value,
child: Text(
value,
style: TextStyle(color: Colors.black),
),
);
}).toList(),
),
],
);
}
}
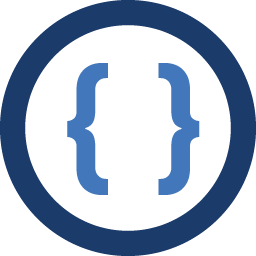
Admin
Updated on December 17, 2022Comments
-
Admin over 1 year
I have an issue with the dropdownbutton in Flutter. To make it quick, I have a list of cities in Cloud Firestore which is used to make the list (items) of the dropdownbutton. This list of cities is getted with a StreamBuilder so when I changed the name of a city, it is changed in real time. The problem here is if I select for exemple 'New York' and in Cloud Firestore I delete 'New York', the dropdownbutton can't find the value 'New York'. I wanted to know if there is a possible way for the dropdownbutton to rebuild and choose a valid value or an other way to manage the deletion of a value in the dropdownbutton ?