How to make a table from a dictionary with multiple content types in Swift?
11,060
You have to implement UITableViewDataSource methods
Remember to set dataSource property of tableView to ViewController
Than you get one object(your NSDictionary) from array and set cell labels and imageView with it's data.
func tableView(tableView: UITableView, numberOfRowsInSection section: Int) -> Int
func tableView(tableView: UITableView, cellForRowAtIndexPath indexPath:NSIndexPath) -> UITableViewCell
Here is full Code example in Swift
. Objective-C is very similar
class MasterViewController: UITableViewController {
var objects = [
["name" : "Item 1", "image": "image1.png"],
["name" : "Item 2", "image": "image2.png"],
["name" : "Item 3", "image": "image3.png"]]
override func tableView(tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return objects.count
}
override func tableView(tableView: UITableView, cellForRowAtIndexPath indexPath: NSIndexPath) -> UITableViewCell {
let cell = tableView.dequeueReusableCellWithIdentifier("Cell", forIndexPath: indexPath) as UITableViewCell
let object = objects[indexPath.row]
cell.textLabel?.text = object["name"]!
cell.imageView?.image = UIImage(named: object["image"]!)
cell.otherLabel?.text = object["otherProperty"]!
return cell
}
}
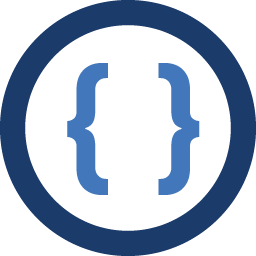
Author by
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
I have made an NSArray with NSDictionary objects containing contents downloaded from an api. I also made a tableview object on main.storyboard with a prototype cell with a UIImage label and two text labels as its contents. How can I put the data from array to table so that each cell with same style as my prototype shows contents of NSDictionary from the array.