How to make buttons span mutiple columns/rows with GridPane JavaFX?
12,018
Since you're using a grid pane, you don't really need the HBox
and VBox
:
import javafx.stage.*;
import javafx.application.*;
import javafx.scene.*;
import javafx.scene.control.*;
import javafx.scene.layout.*;
public class SimpleGUI extends Application {
public void start(Stage primaryStage) throws Exception {
GridPane root = new GridPane();
Scene scene = new Scene(root);
//Buttons
Button b1 = new Button("KNAPP 1");
Button b2 = new Button("KNAPP 2");
Button b3 = new Button("KNAPP 3");
Button b4 = new Button("KNAPP 4");
Button b5 = new Button("KNAPP 5");
Button b6 = new Button("KNAPP 6");
root.add(b1, 0, 0);
root.add(b2, 1, 0);
root.add(b3, 2, 0);
root.add(b4, 0, 1);
root.add(b5, 0, 2);
// node, columnIndex, rowIndex, columnSpan, rowSpan:
root.add(b6, 1, 1, 2, 2);
// allow button to grow:
b6.setMaxSize(Double.MAX_VALUE, Double.MAX_VALUE);
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args){
launch(args);
}
}
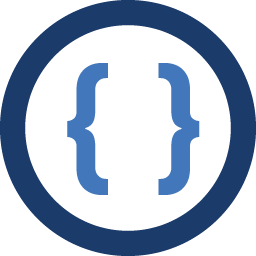
Author by
Admin
Updated on June 12, 2022Comments
-
Admin almost 2 years
I'm new to JavaFX and is trying to make a simple button design with GridPane.
I can't figure out how to make a button span multiple columns/rows without it pushing other buttons out of the way. I've been using HBox and VBox to group the other buttons together. I've tried setRowSpan on the buttons, but that didn't seem to work.
Here is my code:
import javafx.stage.*; import javafx.application.*; import javafx.scene.*; import javafx.scene.control.*; import javafx.scene.layout.*; public class SimpleGUI extends Application { public void start(Stage primaryStage) throws Exception { GridPane root = new GridPane(); Scene scene = new Scene(root, 200, 200); //Buttons Button b1 = new Button("KNAPP 1"); Button b2 = new Button("KNAPP 2"); Button b3 = new Button("KNAPP 3"); Button b4 = new Button("KNAPP 4"); Button b5 = new Button("KNAPP 5"); Button b6 = new Button("KNAPP 6"); //Horizontal Box HBox topButtons = new HBox(); topButtons.getChildren().add(b1); topButtons.getChildren().add(b2); topButtons.getChildren().add(b3); //Vertical Box VBox leftButtons = new VBox(); leftButtons.getChildren().add(b4); leftButtons.getChildren().add(b5); //Placement GridPane.setConstraints(topButtons, 0,0); GridPane.setConstraints(leftButtons, 0,1); GridPane.setConstraints(b6, 1,1); //Length (3 Columns, 2 Rows) GridPane.setColumnSpan(topButtons, 3); GridPane.setRowSpan(leftButtons, 2); //Add them to the stage root.getChildren().add(topButtons); root.getChildren().add(leftButtons); root.getChildren().add(b6); primaryStage.setScene(scene); primaryStage.show(); } public static void main(String[] args){ launch(args); } }