Check which Button object is clicked using JavaFX
13,265
Solution 1
Here is an example:
public class Main extends Application {
private int lastClickedIndex = -1;
@Override
public void start(Stage primaryStage) {
try {
BorderPane root = new BorderPane();
Scene scene = new Scene(root, 400, 400);
Button[] digitButtons = new Button[10];
for(int i = 0; i < 10; i++) {
final int buttonInd = i;
digitButtons[i] = new Button(Integer.toString(i));
digitButtons[i].setOnAction(e -> {
System.out.println("Button pressed " + ((Button) e.getSource()).getText());
lastClickedIndex = buttonInd;
});
}
root.setCenter(new HBox(digitButtons));
primaryStage.setScene(scene);
primaryStage.show();
} catch (Exception e) {
e.printStackTrace();
}
}
public static void main(String[] args) {
launch(args);
}
}
Note: It is unnecessary to get the index as the Button
is exactly specified as the event source: e.getSource()
.
Solution 2
a simple way like
FXML
create 2 buttons call B1
and B2
, and add fx:id
for each button)
<?xml version="1.0" encoding="UTF-8"?>
<?import java.lang.*?>
<?import java.util.*?>
<?import javafx.scene.*?>
<?import javafx.scene.control.*?>
<?import javafx.scene.layout.*?>
<AnchorPane id="AnchorPane" prefHeight="200" prefWidth="320" xmlns:fx="http://javafx.com/fxml/1" xmlns="http://javafx.com/javafx/8" fx:controller="ask.FXMLDocumentController">
<children>
<Button fx:id="B1" layoutX="94.0" layoutY="106.0" text="B1" />
<Button fx:id="B2" layoutX="195.0" layoutY="106.0" text="B2" />
</children>
</AnchorPane>
Controller
use setOnAction
method to change value
Button name must same as what fx:id set
import java.net.URL;
import java.util.ResourceBundle;
import javafx.fxml.FXML;
import javafx.fxml.Initializable;
import javafx.scene.control.Button;
public class FXMLDocumentController implements Initializable {
@FXML Button B1;
@FXML Button B2;
int whichIsLastClicked = -1;
public void initialize(URL url, ResourceBundle rb)
{
B1.setOnAction(e->whichIsLastClicked=1);
B2.setOnAction(e->whichIsLastClicked=2);
}
}
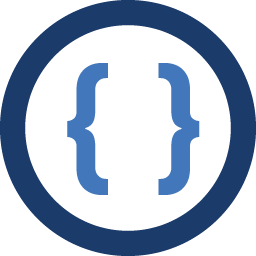
Author by
Admin
Updated on June 15, 2022Comments
-
Admin almost 2 years
I am making a basic calculator but I am unsure of how to check if a certain
Button
has been clicked. I want to use an array of Button objects and then check to see if the button clicked matches a button object in the array. My code for this is below.Button[] digitButtons = { bt0, bt1, bt2, bt3, bt4, bt5, bt6, bt7, bt8, bt9 }; for (int index = 0; index < 9; index++) { int position = -1; if (buttonClicked == digitButtons[index]) { position = index; break; } }