How to make everything in a string lowercase
Solution 1
As per official documentation,
str.lower()
Return a copy of the string with all the cased characters [4] converted to lowercase.
So you could use it at several different places, e.g.
lines.append(line.lower())
reversed_line = remove_punctuation(line).strip().split(" ").lower()
or
print(len(lines) - i, " ".join(reversed_line).lower())
(this would not store the result, but only print it, so it is likely not what you want).
Note that, depending on the language of the source, you may need a little caution, e.g., this. See also other relevant answers for How to convert string to lowercase in Python
Solution 2
I think changing the second to last line to this may work
print(len(lines) - i, " ".join(reversed_line).lower())
Solution 3
Store the contents of a file in a variable, the assign it to itself .lower() like so:
fileContents = inputFile.readline()
fileContents = fileContents.lower()
Solution 4
You could probably insert it here, for instance:
lines.append(line.lower())
Note that line.lower()
does not do anything to line
itself (strings are immutable!), but returns a new string object. To make line hold that lowercase string, you'd do:
line = line.lower()
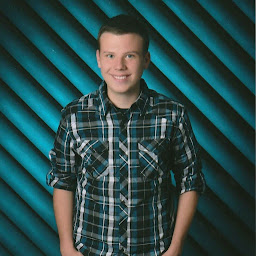
Alex Bass
Updated on November 01, 2020Comments
-
Alex Bass over 3 years
I am trying to write a function that will print a poem reading the words backwards and make all the characters lower case. I have looked around and found that .lower() should make everything in the string lowercase; however I cannot seem to make it work with my function. I don't know if I'm putting it in the wrong spot or if .lower() will not work in my code. Any feedback is appreciated!
Below is my code before entering .lower() anywhere into it:
def readingWordsBackwards( poemFileName ): inputFile = open(poemFileName, 'r') poemTitle = inputFile.readline().strip() poemAuthor = inputFile.readline().strip() inputFile.readline() print ("\t You have to write the readingWordsBackwards function \n") lines = [] for line in inputFile: lines.append(line) lines.reverse() for i, line in enumerate(lines): reversed_line = remove_punctuation(line).strip().split(" ") reversed_line.reverse() print(len(lines) - i, " ".join(reversed_line)) inputFile.close()