How to make some columns align left and some column align center in React Table - React
Method 1:
Something like this should do the job
columns: [
{
accessor: "firstName",
Header: () => (
<div
style={{
textAlign:"right"
}}
>S Column 1</div>)
},
If you can help me, please provide a demo on CodeSandbox
Play here
Update after OP comment
but for columns which will be centre aligned , their sub cell data will also be centre aligned
Manipulate cell styles like this
Cell: row => <div style={{ textAlign: "center" }}>{row.value}</div>
Play it here
Method 2:
Use can use the headerClassName
and specify a class name, in that class you can specify the rule text-align:center
So the code will look like
const columns = [{
Header: 'Column 5',
accessor: 'name',
headerClassName: 'your-class-name'
},
{
......
}
]
Method 3:
If you have lot of headers, adding headerClassName in all headers is a pain. In that case you can set the class name in ReactTableDefaults
import ReactTable, {ReactTableDefaults} from 'react-table';
const columnDefaults = {...ReactTableDefaults.column, headerClassName: 'text-left-classname'}
and in the ReactTable, use like this
<ReactTable
...
...
column = { columnDefaults }
/>
Note: if you are using bootstrap you can assign the inbuilt class
text-center
toheaderClassName
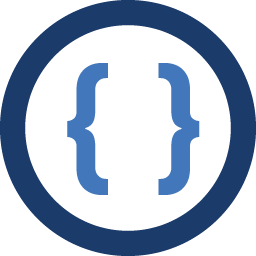
Admin
Updated on September 18, 2021Comments
-
Admin almost 3 years
Hello Stack overflow members
This is array for the column headers. I want column 1 to column 5 left align (all the header, sub header and table data cells of column 1 to column 5 to be left aligned) while I I want column 6 to column 8 to be centre aligned (all the header, sub header and table data cells of column 1 to column 5 to be centre aligned). Please help me to solve this as I can only make either all columns center or all columns left aligned.
I want to implement this particular style on column 6 to 8 header as shown in this image.
.
If you can help me, please provide a demo on CodeSandbox
This is my Header Data
const columns = [ { Header: 'Column 1', columns: [ { Header: 'S Column 1', accessor: 'firstName' } ] }, { Header: 'Column 2', columns: [ { Header: 'S Column 2', accessor: 'firstName' } ] }, { Header: 'Column 3', columns: [ { Header: 'S Column 3', accessor: 'firstName' } ] }, { Header: 'Column 4', columns: [ { Header: 'S column 4', accessor: 'firstName' } ] }, { Header: 'Column 5', columns: [ { Header: 'S column 5', accessor: 'firstName' } ] }, { Header: 'Column 6', columns: [ { Header: 'S column 6a', accessor: 'firstName' }, { Header: 'S column 6b', accessor: 'firstName' }, { Header: 'S column 6c', accessor: 'firstName' }, { Header: 'S column 6d', accessor: 'firstName' } ] }, { Header: 'Column 7', columns: [ { Header: 'S column 7', accessor: 'firstName' } ] }, { Header: 'Column 8', columns: [ { Header: 'S Column 8a', accessor: 'firstName' }, { Header: 'S Column 8b', accessor: 'firstName' }, { Header: 'S Column 8c', accessor: 'firstName' }, { Header: 'S Column 8d', accessor: 'firstName' } ] }, ];