How to open a jdialog from a jframe
The constructor on JDialog accept parent object. But you use new JFrame object. This is error (logical error).
AddClient dialog = new AddClient(MainWindow.this);
If you want modal dialog, call
dialog.setModal(true);
And set default close operation.
dialog.setDefaultCloseOperation(JDialog.DISPOSE_ON_CLOSE);
Use of thread is unnecessary.
Ps: sorry for bad English
Edit: Here is simple example with exit code in dialog
public class AddClient extends JDialog {
public static final int ID_OK = 1;
public static final int ID_CANCEL = 0;
private int exitCode = ID_CANCEL;
public AddClient(Frame owner) {
super(owner);
createGUI();
}
public AddClient(Dialog owner) {
super(owner);
createGUI();
}
private void createGUI() {
setPreferredSize(new Dimension(600, 400));
setTitle(getClass().getSimpleName());
pack();
setLocationRelativeTo(getParent());
}
@Override
public void dispose() {
super.dispose();
}
public int doModal() {
setDefaultCloseOperation(JDialog.DISPOSE_ON_CLOSE);
setModal(true);
setVisible(true);
return exitCode;
}
}
And MainWindow
public class MainWindow extends JFrame {
public MainWindow() throws HeadlessException {
super();
createGUI();
}
private void createGUI() {
setExtendedState(JFrame.MAXIMIZED_BOTH);
setDefaultCloseOperation(JFrame.DISPOSE_ON_CLOSE);
setTitle(getClass().getSimpleName());
JButton addClientButton = new JButton("Add client");
addClientButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
SwingUtilities.invokeLater(new Runnable() {
@Override
public void run() {
AddClient dialog = new AddClient(MainWindow.this);
if(dialog.doModal() == AddClient.ID_OK) {
}
}
});
}
});
JToolBar toolBar = new JToolBar();
toolBar.add(addClientButton);
add(toolBar, BorderLayout.PAGE_START);
}
@Override
public void dispose() {
super.dispose();
}
}
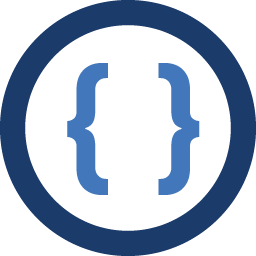
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
JFrame button to open the jdialog:
private void jButton1ActionPerformed(java.awt.event.ActionEvent evt) { // TODO add your handling code here:
JDialog method that opens the dialog:
public void run() { AddClient dialog = new AddClient(new javax.swing.JFrame(), true); dialog.addWindowListener(new java.awt.event.WindowAdapter() { @Override public void windowClosing(java.awt.event.WindowEvent e) { System.exit(0); } }); dialog.setVisible(true);
My JDialog class is named AddClient, my JFrame class is named MainWindow. The package these are in is named my.javaapp
How would i go onto doing this? I'm pretty new to java, was using python up until this point and dove head in into java swing gui builder with netbeans, i'm planning on watching tutorials and reading through the java documentation to learn more about java, but i would like to start by being able to open this jdialog.
Before asking this question i searched a lot, and i read other questions that had been asked to solve more or less this same problem, i still couldn't solve mine though so i'm asking.
Solution:
Delete the main function from the class the gui you want to pop up is on e.g
public static void main(String args[]) { try { for (javax.swing.UIManager.LookAndFeelInfo info : javax.swing.UIManager.getInstalledLookAndFeels()) { if ("Nimbus".equals(info.getName())) { javax.swing.UIManager.setLookAndFeel(info.getClassName()); break; } } } catch (ClassNotFoundException ex) { java.util.logging.Logger.getLogger(NewJDialog1.class.getName()).log(java.util.logging.Level.SEVERE, null, ex); } catch (InstantiationException ex) { java.util.logging.Logger.getLogger(NewJDialog1.class.getName()).log(java.util.logging.Level.SEVERE, null, ex); } catch (IllegalAccessException ex) { java.util.logging.Logger.getLogger(NewJDialog1.class.getName()).log(java.util.logging.Level.SEVERE, null, ex); } catch (javax.swing.UnsupportedLookAndFeelException ex) { java.util.logging.Logger.getLogger(NewJDialog1.class.getName()).log(java.util.logging.Level.SEVERE, null, ex); } //</editor-fold> /* Create and display the dialog */ java.awt.EventQueue.invokeLater(new Runnable() { public void run() { NewJDialog1 dialog = new NewJDialog1(new javax.swing.JFrame(), true); dialog.addWindowListener(new java.awt.event.WindowAdapter() { @Override public void windowClosing(java.awt.event.WindowEvent e) { System.exit(0); } }); dialog.setVisible(true); } }); }
Before deleting though, copy this line:
NewJDialog1 dialog = new NewJDialog1(new javax.swing.JFrame(), true);
Right click the button you want to pop this window up after pressing, events > action > action performed. Paste it there like this:
private void jButton2ActionPerformed(java.awt.event.ActionEvent evt) { NewJDialog1 dialog = new NewJDialog1(new javax.swing.JFrame(), true); dialog.setVisible(true); }
Remember to add the last line, that's what shows up the dialog.