How to organize material-ui Grid into rows?
Solution 1
You are close with the second block of code.
I found that you could simply create 2 distinct Grid sections such as:
<div>
<Grid id="top-row" container spacing={24}>
<Grid item xs={4}>
<Paper className={classes.paper}>Grid cell 1, 1</Paper>
</Grid>
<Grid item xs={4}>
<Paper className={classes.paper}>Grid cell 2, 1</Paper>
</Grid>
</Grid>
<Grid id="bottom-row" container spacing={24}>
<Grid item xs={4}>
<Paper className={classes.paper}>Grid cell 1, 2</Paper>
</Grid>
<Grid item xs={4}>
<Paper className={classes.paper}>Grid cell 2, 2</Paper>
</Grid>
</Grid>
</div>
You can play with it in my sandbox
It might also be work checking out the official documentation for Grid, as it shows a few ways to use it and also links to each exapmle hosted on codesandbox.io so you can play with it yourself.
From the docs, it seems the best way to have multi-layered grid systems is to define the width of the overall grid and then to define the width of each cell, as this will push cells later in the series onto the other rows.
Solution 2
Here's another approach you could take which does not require two grid containers. In Material UI you can organise a grid into rows by using the fact that a row has 12 columns and making use of empty grid items (as Material UI does not support offset grid items) e.g.
<Grid container spacing={24}>
<Grid item xs={4}>
<Paper className={classes.paper}>Grid cell 1, 1</Paper>
</Grid>
<Grid item xs={4}>
<Paper className={classes.paper}>Grid cell 2, 1</Paper>
</Grid>
<Grid item xs={4} />
{/* 12 Columns used, so next grid items will be the next row */}
{/* This works because Material UI uses Flex Box and flex-wrap by default */}
<Grid item xs={4}>
<Paper className={classes.paper}>Grid cell 1, 2</Paper>
</Grid>
<Grid item xs={8} />
</Grid>
You can see this working here.
Indeed this is actually demonstrated on the Material UI websites (though without the offsets) here.
I admit though that I'd prefer to see semantic Row and Column elements in Material UI (e.g. like in Bootstrap), but this is not how it works.
Solution 3
You can do something like this
<Grid container spacing={1}>
<Grid container item xs={12} spacing={3}>
<Grid item xs={4}>
<Paper className={classes.paper}>item</Paper>
</Grid>
</Grid>
<Grid container item xs={12} spacing={3}>
<Grid item xs={4}>
<Paper className={classes.paper}>item</Paper>
</Grid>
</Grid>
<Grid container item xs={12} spacing={3}>
<Grid item xs={4}>
<Paper className={classes.paper}>item</Paper>
</Grid>
</Grid>
</Grid>
Solution 4
I created new component to solve this problem:
export const GridBreak = styled.div`
width: 100%
`;
Then your code would look like this:
<Grid container>
<Grid item xs={4} />
<Grid item xs={4} />
<GridBreak />
<Grid item xs={4} />
</Grid>
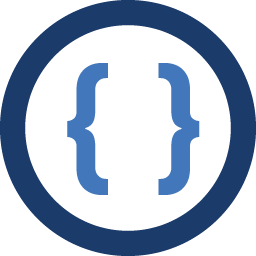
Admin
Updated on March 07, 2021Comments
-
Admin about 3 years
I'm using material-ui to do a form, using the
Grid
system I'd like to do the following:<Grid container> <Grid item xs={4} /> <Grid item xs={4} /> <Grid item xs={4} /> </Grid>
And be able to put the first 2 items, on the first row and the third on a second row, the only way I found to do it is:
<Grid container> <Grid item xs={4} /> <Grid item xs={4} /> </Grid> <Grid container> <Grid item xs={4} /> </Grid>
What is the better way to stack material-ui
Grid
into rows (like the row class concept in Bootstrap grid)?I also thought about these options:
filling the first row with empty
Grid
item?putting vertical container?