How to override a previously set jquery event handler?
Solution 1
Yes, just use .off()
, like
$('selector').off('eventname')
Solution 2
This is a bit of a hack, but it sounds like you're trying to hack on someone else's code without the ability to change it directly so that may be what you have to resort to.
If you just need to call something after their document.ready()
and you don't control the order of the document.ready()
statements, then you can put some code in a short timeout like this inside your document.ready handler:
$(document).ready(function() {
setTimeout(function() {
$('selector').off('eventname').on(your event handler here);
}, 1);
});
The setTimeout()
will run AFTER all document.ready() handlers have run.
Solution 3
Another way:
$(window).on('load', function() {
$('selector').off('eventname').on(your event handler here);
});
Load is executed after document ready
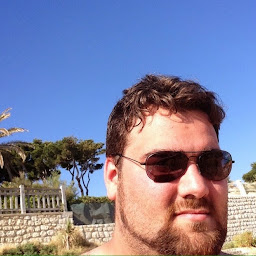
Comments
-
gregory boero.teyssier almost 2 years
In my code, an event handler for an element is set which changes that element's css height to 100px. At somewhere else, I want a different event handler to be run if certain conditions meet, which should override the previous event handler and change its height to 200px.
Is there a way to do that, or to clear all previously set event handlers for an element?
-
gregory boero.teyssier over 11 yearsIs there any code to check if any events have been set for the given element or not. So I could do:
if ( $(ele).hasHandler('click') ) /*clear event/* else setTimeout(this, 1000);
, to make sure that the event has been set before my function is called -
jfriend00 over 11 years@ClickUpvote - there's no need to check first. Just remove any previously applied handlers, then attach your own. If there were none, removing will just do nothing. FYI, this ONLY works for jQuery applied event handlers, not plain javascript applied handlers.
-
gregory boero.teyssier over 11 yearsRight, but if I could check before removing, that would work more reliably, e.g check if event is set, if yes, remove & set new, if not, check again 1 sec later, repeat
-
jfriend00 over 11 yearsUnless the other code is also setting the event handler on a timer (in which case now you're really doing a hack), there is no need for multiple checks/timers. If it's set on document.ready, then your timer set from document.ready will be AFTER all document.ready handlers.
-
gregory boero.teyssier over 11 yearscan you just answer the question? :)
-
jfriend00 over 11 years@ClickUpvote - I don't know the answer. Somewhere buried in jQuery is a way to get at other jQuery event handlers, but I don't know it personally. You will have to find it. My recommendation is that it is not needed and there is probably a much cleaner way to solve the issue, but you've not disclosed much of the problem and already selected a best answer so apparently your original question is already answered to your satisfaction.
-
zerkms over 11 years@ClickUpvote: you can do that using something like this: jsfiddle.net/zerkms/7w5Xk jQuery source reference: github.com/jquery/jquery/blob/master/src/event.js#L20
-
Saitama about 6 yearsNice! Good job haha