How to plot a graph from csv in python
11,810
Solution 1
import matplotlib.pyplot as plt
import pandas as pd
%matplotlib inline # jupyter notebook
# Load data
data = pd.read_csv('your_csv_file.csv')
# Plot
plt.figure(figsize=(6.8, 4.2))
x = range(len(data['month']))
plt.plot(x, data['sales'])
plt.xticks(x, data['month'])
plt.xlabel('Month')
plt.ylabel('Sales')
plt.show()
I hope this will help you.
Solution 2
df =pd.read_csv('filename.csv', sep=',')
months = {'jan':1,
'feb':2,
'mar':3,
'apr':4,
'may':5,
'jun':6,
'jul':7,
'aug':8,
'sep':9,
'oct':10,
'nov':11,
'dec':12
}
plt.plot(df['month'].replace(months), df['sales'], label='sales')
plt.plot(df['month'].replace(months), df['expenditure'], label='expenditure')
plt.gca().set_xticks(list(months.values()))
plt.gca().set_xticklabels(list(months.keys()))
plt.legend()
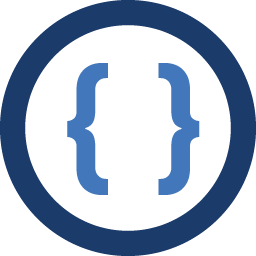
Author by
Admin
Updated on June 27, 2022Comments
-
Admin almost 2 years
I have the following code and was wondering how to plot it as a graph in python
year,month,sales,expenditure 2018,jan,6226,3808 2018,feb,1521,3373 2018,mar,1842,3965 2018,apr,2051,1098 2018,may,1728,3046 2018,jun,2138,2258 2018,jul,7479,2084 2018,aug,4434,2799 2018,sep,3615,1649 2018,oct,5472,1116 2018,nov,7224,1431 2018,dec,1812,3532
this is my code so far
import matplotlib.pyplot as plt import csv x = [] y = [] with open('sales.csv','r') as sales_csv: plots = csv.reader(sales_csv, delimiter=',') for row in plots: x.append(row[1]) y.append(row[3]) plt.plot(x,y, label='Loaded from file!') plt.xlabel('x') plt.ylabel('y') plt.show()
-
Admin almost 4 yearsWhat would the entire code look like? The above doesn't seem to work for me
-
warped almost 4 yearswhat does "doesn't seem to work" mean? Do you get an error message?
-
Admin almost 4 yearsNo worries - I worked it out! I didn't include the file name in - Thank you for your help!!