How to prevent caching images from network flutter?
2,337
Solution 1
I have just created a widget that gets the image as a URL and then download it as Uint8List
and show it in a Image.memory
widget.
You can use it like this:
NonCacheNetworkImage('https://riverpod.dev/img/logo.png'),
import 'dart:typed_data';
import 'package:flutter/material.dart';
import 'package:http/http.dart';
class NonCacheNetworkImage extends StatelessWidget {
const NonCacheNetworkImage(this.imageUrl, {Key? key}) : super(key: key);
final String imageUrl;
Future<Uint8List> getImageBytes() async {
Response response = await get(Uri.parse(imageUrl));
return response.bodyBytes;
}
@override
Widget build(BuildContext context) {
return FutureBuilder<Uint8List>(
future: getImageBytes(),
builder: (context, snapshot) {
if (snapshot.hasData) return Image.memory(snapshot.data!);
return SizedBox(
width: 100,
height: 100,
child: Text("NO DATA"),
);
},
);
}
}
Solution 2
You can achieve that simply by adding ?
followed by timestamp
at the end of your image url.
Image.network(
'${imgUrl}?${DateTime.now().millisecondsSinceEpoch.toString()}',
fit: BoxFit.cover,
),
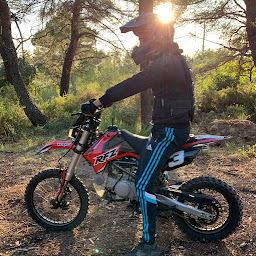
Author by
Tom3652
Updated on December 31, 2022Comments
-
Tom3652 over 1 year
I have tried all of the following
Widget
to load images from network :Image.network()
CachedNetworkImage()
And also their
ImageProvider
:NetworkImage
CachedNetworkImageProvider
There is no
bool
to choose not to cache images. The only way i have found is to load theImageProvider
like ininitState()
and then callevict()
right after.I don't really know if this works actually or if this is the best way to do...
Is there any way to prevent caching from network "natively" ?
-
Benyamin almost 3 yearsImage.network() does not cache the image. it downloads it again every time u go to widget.
-
Tom3652 almost 3 yearsfrom the documentation api.flutter.dev/flutter/widgets/Image/Image.network.html :
All network images are cached regardless of HTTP headers.
. Am i not understanding this sentence correctly ? -
Benyamin almost 3 yearsI guess you are right. reference here stackoverflow.com/questions/47209606/… I think this is your answer
-
Tom3652 almost 3 yearsthank you for the link, but does it mean that every instance of ImageCache will be cleared automatically ? Meaning that everytime we load an ImageProvider it will be evicted from cache ?
-
Yeasin Sheikh almost 3 yearsWhere are you testing/ web does cache automatically. But on android/ios it doesn't
-
Tom3652 almost 3 yearsYes it is Android / IOS only. and you are right i have the output that there is no "pending" cache configuration for a given provider.