How to prevent multiple event on same UIButton in iOS?
12,300
Solution 1
What you're doing is, you simply setting enabled on/off outside of the block. This is wrong, its executing once this method will call, thus its not disabling the button until completion block would call. Instead you should reenable it once your animation would get complete.
-(IBAction) buttonClick:(id)sender{
button.enabled = false;
[UIView animateWithDuration:1.0 delay:0.0 options:UIViewAnimationOptionAllowAnimatedContent animations:^{
// code to execute
}
completion:^(BOOL finished){
// code to execute
button.enabled = true; //This is correct.
}];
//button.enabled = true; //This is wrong.
}
Oh and yes, instead of true
and false
, YES
and NO
would looks nice. :)
Solution 2
Instead of using UIView animation I decided to use the Timer
class to enable the button after a time interval. Here is the answer using Swift 4:
@IBAction func didTouchButton(_ sender: UIButton) {
sender.isUserInteractionEnabled = false
//Execute your code here
Timer.scheduledTimer(withTimeInterval: 2, repeats: false, block: { [weak sender] timer in
sender?.isUserInteractionEnabled = true
})
}
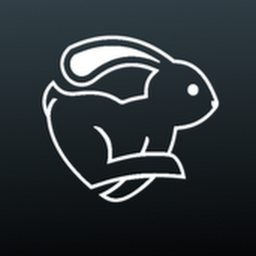
Author by
crazydev
Updated on July 24, 2022Comments
-
crazydev almost 2 years
I want to prevent continuous multiple clicks on the same
UIButton
.I tried with
enabled
andexclusiveTouch
properties but it didn't work. Such as:-(IBAction) buttonClick:(id)sender{ button.enabled = false; [UIView animateWithDuration:1.0 delay:0.0 options:UIViewAnimationOptionAllowAnimatedContent animations:^{ // code to execute } completion:^(BOOL finished){ // code to execute }]; button.enabled = true; }