How to print the results of a split in Java
10,002
Solution 1
You can use Arrays.toString
to print the String representation of your array: -
System.out.println(Arrays.toString(b);
This will print your array like this: -
[A, b, C ]
Or, if you want to print each element separately without that square brackets
at the ends, you can use enhanced for-loop
: -
for(String val: b) {
System.out.print(val + " ");
}
This will print your array like this: -
A b C
Solution 2
If you want each element printed on a separate line, you can do this:
public class Testing {
public static void main (String [] args){
String a = "A#b#C ";
String[] b = a.split("#");
for (String s : b) {
System.out.println(s);
}
}
}
For a result like [A, b, C]
, use Rohit's answer.
Solution 3
Please try this
String a = "A#b#C ";
String[] b = a.split("#");
for( int i = 0; i < b.length; i++)
{
System.out.println(b[i]);
}
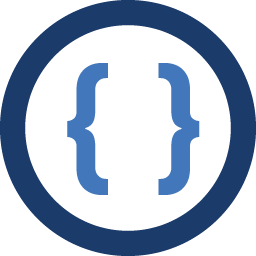
Author by
Admin
Updated on June 14, 2022Comments
-
Admin almost 2 years
How do I make this print the contents of b rather than its memory address?
public class Testing { public static void main (String [] args){ String a = "A#b#C "; String[] b = a.split("#"); System.out.println(b); } }
-
Ted Hopp over 11 yearsThat won't print the last element. You need
b.length
instead ofb.length - 1
(or else<=
instead of<
). -
Ted Hopp over 11 yearsDid you try using
asList
? I don't think it does what you think. -
Sumit Singh over 11 yearsYes it will return List and if you print it then it will print all the element because of lists toString method.
-
Ted Hopp over 11 yearsThere is no
toString()
method specified in theList
interface. Your code only works because theList
thatArrays.toList
returns happens to be a subclass ofAbstractCollection
, which does implementtoString
the way you want. There's nothing in the specification ofArrays.asList
that requires that the returned list be a subclass ofAbstractCollection
or that theList
that is returned override thetoString
method inherited fromObject
.