How to re-position Google Maps logo and map buttons
Solution 1
I found a workaround, not the best solution I guess, but should work
simply add padding to GoogleMap() widget, set bottom and left values as your wish
new GoogleMap(
padding: EdgeInsets.only(bottom: 100, left: 15), // <--- padding added here
// rest of parameters
// ....
)
NOTE !!
If you pick locations from a point at the center of the screen, adding padding to GoogleMap() should affect/change the values of location picked. so have that considered.
Thank you
Solution 2
Apparently there is no direct/reliable way to do so and even if you can somehow use Stack to cover that logo you shouldn't do it either.
You might get into trouble while uploading your app on Google Playstore.
If you don't care about moral values just read through this:
9.4 Attribution.
(a) Content provided to you through the Service may contain the trade names, trademarks, service marks, logos, domain names, and other distinctive brand features of Google, its partners, or other third party rights holders of content indexed by Google. When Google provides this attribution, you must display it as provided through the Service or as described in the Maps APIs Documentation and may not delete or in any manner alter these trade names, trademarks, service marks, logos, domain names, and other distinctive brand features.
https://developers.google.com/maps/terms
Google already offers a -WIDE- range of free and freemium services from fonts to ... to ... to Flutter. Imagine if you were instead of Google what would you do if you find someone trying to misuse your app's free service which actually costs you money.
If you just want to relocate the icon edit the source code of that package by hovering on GoogleMap() while pressing Ctrl and then right click when the text turns blue. A new window with source code of GoogleMap widget will appear where you can check and edit how the position of the logo is specified but I can't guarantee you that it is allowed.
Solution 3
Google has already simplified this for you, adapting from Rami answer, you can simply do the following to add padding to your map
GoogleMap(
myLocationEnabled: true,
padding: EdgeInsets.only(bottom: mapBottomPadding, top: 0, right: 0, left: 0),
myLocationButtonEnabled: true,
compassEnabled: true,
mapToolbarEnabled: true,
mapType: MapType.normal,
markers: _markers,
initialCameraPosition: _kLake,
onMapCreated: (GoogleMapController controller) {
_controller.complete(controller);
mapController = controller;
setState(() {
mapBottomPadding = 280;
_goToTheLake();
});
},
),
The map camera target is adjusted accordingly based on the padding applied to the map either to the left, top, right or bottom.
Hope this helps.
Solution 4
As mentioned by this GitHub issue (open as of July 22, 2020), you must use a StatefulWidget
and setState()
method when setting padding for the Google Maps logo (or other map buttons). Without calling setState()
, the padding will not properly apply on Android. This seems to be an issue with the native Android Maps SDK, as the same padding issue occurs in React Native's Google Maps package.
As per Google Maps Platform Terms of Service, you must display the Google logo on your Google Map at all times, so you cannot remove it without a workaround. However, you can remove other buttons on the Google Map, like the location and zoom in/out buttons.
See the example below for an implementation of padding on the Google Maps logo, as well as removing the location and zoom in/out buttons:
GoogleMap(
//padding parameter for positioning the Google logo and other map buttons
padding: EdgeInsets.only(
bottom: 120,
),
//remove zoom in/out buttons
zoomControlsEnabled: false,
//remove location button
myLocationButtonEnabled: false,
initialCameraPosition: CameraPosition(
target: _center,
zoom: 12.0,
),
onMapCreated: (GoogleMapController controller) {
//required to apply padding correctly on Android
setState(() {});
},
)
To find more info on on parameters of the GoogleMap
widget, view the google_maps_flutter package documentation .
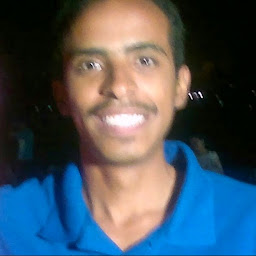
Rami Ibrahim
Updated on December 13, 2022Comments
-
Rami Ibrahim over 1 year
How do you change the position of the Google logo (originally at the bottom left corner) and other map buttons (location, zoom in/out, etc.) of the GoogleMap widget?
I would also like to know if you can disable/enable them.
I am using google_maps_flutter package version
^0.5.19
.