how to read a text file in windows universal app
Solution 1
the code is very simple, you just have to use a valid scheme URI (ms-appx in your case) and transform your WinRT InputStream as a classic .NET stream :
var file = await StorageFile.GetFileFromApplicationUriAsync(new Uri("ms-appx:///thedata.txt"));
using (var inputStream = await file.OpenReadAsync())
using (var classicStream = inputStream.AsStreamForRead())
using (var streamReader = new StreamReader(classicStream))
{
while (streamReader.Peek() >= 0)
{
Debug.WriteLine(string.Format("the line is {0}", streamReader.ReadLine()));
}
}
For the properties of the embedded file, "Build Action" must be set to "Content" and "Copy to Ouput Directory" should be set to "Do not Copy".
Solution 2
You can't use classic .NET IO methods in Windows Runtime apps, the proper way to read a text file in UWP is:
var file = await ApplicationData.Current.LocalFolder.GetFileAsync("data.txt");
var lines = await FileIO.ReadLinesAsync(file);
Also, you don't need a physical path of a folder - from msdn :
Don't rely on this property to access a folder, because a file system path is not available for some folders. For example, in the following cases, the folder may not have a file system path, or the file system path may not be available. •The folder represents a container for a group of files (for example, the return value from some overloads of the GetFoldersAsync method) instead of an actual folder in the file system. •The folder is backed by a URI. •The folder was picked by using a file picker.
Solution 3
Please refer File access permissions for more details. And Create, write, and read a file provides examples related with File IO for UWP apps on Windows 10.
You can retrieve a file directly from your app's local folder by using an app URI, like this:
using Windows.Storage;
StorageFile file = await StorageFile.GetFileFromApplicationUriAsync("ms-appdata:///local/file.txt");
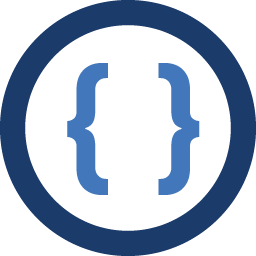
Admin
Updated on June 16, 2020Comments
-
Admin almost 4 years
I am trying to read a text file named thedata.txt that has a list of words that I want to use in a hangman game. I have tried different ways, but I can't figure out where the file gets placed, if at all when the app runs. I added the file to my project, and I have tried setting the build properties to content, and then embedded resource, but can't find the file. I have made a Windows 10 universal app project. The code I tried looks like this:
Stream stream = this.GetType().GetTypeInfo().Assembly.GetManifestResourceStream("thedata.txt"); using (StreamReader inputStream = new StreamReader(stream)) { while (inputStream.Peek() >= 0) { Debug.WriteLine("the line is ", inputStream.ReadLine()); } }
I get exceptions. I also tried to list the files in another directory:
string path = Windows.Storage.ApplicationData.Current.LocalFolder.Path; Debug.WriteLine("The path is " + path); IReadOnlyCollection<StorageFile> files = await Windows.Storage.ApplicationData.Current.LocalFolder.GetFilesAsync(); foreach (StorageFile file2 in files) { Debug.WriteLine("Name 2 is " + file2.Name + ", " + file2.DateCreated); }
I don't see the file there either...I want to avoid hard coding the list of names in my program. I'm not sure what the path that the file is placed.
-
Admin over 8 yearsThe above code provided by t.ouvre answered my question. I have verified that it works on my computer and my emulator. Thank you for answering my question.
-
Mario Murrent over 8 yearsWorks like a charm. Thanks