how to read lists in yaml?
16,937
Solution 1
I had to rewrite your yaml a bit ...
- !!com.example.Contact
name: Nathan Sweet
age: 28
address: pak
phoneNumbers:
- !!com.example.Phone
name: Home
number: 1122
- !!com.example.Phone
name: Work
number: 3322
- !!com.example.Contact
name: Nathan Sw1eet
age: 281
address: pak1
phoneNumbers:
- !!com.example.Phone
name: Home1
number: 11221
- !!com.example.Phone
name: Work1
number: 33211
Java ...
package com.example;
import org.springframework.core.io.DefaultResourceLoader;
import org.springframework.core.io.Resource;
import org.yaml.snakeyaml.Yaml;
import org.yaml.snakeyaml.constructor.Constructor;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.util.Collection;
public class LoadContacts {
private static String yamlLocation="classpath:contacts.yaml";
public static void main(String[] args) throws IOException{
Yaml yaml = new Yaml(new Constructor(Collection.class));
InputStream in = null;
Collection<Contact> contacts;
try {
in = new FileInputStream(new File(yamlLocation));
contacts = (Collection<Contact>) yaml.load(in);
} catch (IOException e) {
final DefaultResourceLoader loader = new DefaultResourceLoader();
final Resource resource = loader.getResource(yamlLocation);
in = resource.getInputStream();
contacts = (Collection<Contact>) yaml.load(in);
} finally {
if (in != null) {
try {
in.close();
} catch (Exception e) {
// no-op
}
}
}
for(Contact contact:contacts){
System.out.println(contact.name + ":" + contact.address + ":" + contact.age );
}
}
}
Solution 2
Have you tried SnakeYaml
Solution 3
Since I came across this post to read a list of User objects, here is what I did using Jackson
import com.fasterxml.jackson.core.type.TypeReference;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.dataformat.yaml.YAMLFactory;
import java.io.File;
import java.io.IOException;
import java.util.List;
public class LoadContacts {
private static String yamlLocation = "path_to/../contacts.yml";
public static void main(String[] args) throws IOException {
ObjectMapper mapper = new ObjectMapper(new YAMLFactory());
try {
List<Contact> contactList = mapper.readValue(new File(yamlLocation), new TypeReference<List<Contact>>(){});
contactList.forEach(System.out::println);
} catch (Exception e) {
e.printStackTrace();
}
}
}
mapper.readValue(..)
takes in multiple arguments such as URL, String for the first param. This solution uses File
.
One change I did for the OP problem to work correctly was to define phoneNumbers as follows:
public List<Phone> phoneNumbers;
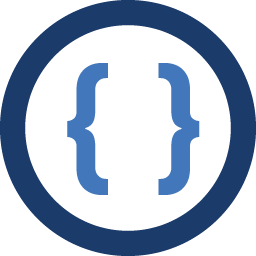
Author by
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
I am trying to read inputs from a yaml file, having the following content:
- !com.example.Contact name: Nathan Sweet age: 28 address: pak phoneNumbers: - !com.example.Phone name: Home number: 1122 - !com.example.Phone name: Work number: 3322 - !com.example.Contact name: Nathan Sw1eet age: 281 address: pak1 phoneNumbers: - !com.example.Phone name: Home1 number: 11221 - !com.example.Phone name: Work1 number: 33211
I have the following defined:
import java.util.List; import java.util.Map; public class Contact { public String name; public int age; public String address; public List phoneNumbers; } public class Phone { public String name; public String number; }
Can some tell me the way to read these phone numbers