Java try catch not handling IndexOutOfBoundsException
11,373
Solution 1
do {
for (int i = 0; i < list.size(); i++) {
System.out.print("(" + (i + 1) + ")" + list.get(i));
}
System.out.println(" ");
try {
option = sc.nextInt();
} catch (IndexOutOfBoundsException e) {
System.out.println("Invalid option");
sc.next();
continue;
} catch (InputMismatchException e) {
System.out.println("Option input mismatch.");
sc.next();
continue;
}
sc.nextLine();
if (option == 1) {
System.out.print("Enter name: ");
// scanner takes in input
} else if (option == 2) {
System.out.print("Enter desc: ");
// scanner takes in input
}
try {
type = list.get((option - 1));
} catch (IndexOutOfBoundsException e) {
System.out.println("Invalid option");
option=3;
}
} while (option <= 0 || option >= 3);
I have added new try-catch at type = list.get((option - 1)); To force user re-input option, I will set option to 3 at the catch cause
Solution 2
You are not going to catch the exception if you don't use an invalid value to call the list.
ArrayList<String> list = new ArrayList<>(Arrays.asList("item1", "item2"));
Scanner sc = new Scanner(System.in);
int option;
try {
option = sc.nextInt();
System.out.println(list.get(option));
} catch (IndexOutOfBoundsException e) {
System.out.println("Invalid option");
} catch (InputMismatchException e) {
System.out.println("Option input mismatch.");
}
sc.close();
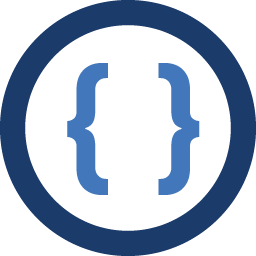
Author by
Admin
Updated on June 14, 2022Comments
-
Admin almost 2 years
I was having some problem when try to try catch the IndexOutOfBoundsException for a List in Java. So I declared my list with 2 elements as:
List<String> list = new ArrayList<>(Arrays.asList("item1", "item2"));
Then I tried to do a try catch:
do { for (int i = 0; i < list.size(); i++) { System.out.print("(" + (i + 1) + ")" + list.get(i)); } System.out.println(" "); try{ option = sc.nextInt(); } catch (IndexOutOfBoundsException e){ System.out.println("Invalid option"); sc.next(); continue; } catch (InputMismatchException e) { System.out.println("Option input mismatch."); sc.next(); continue; } sc.nextLine(); if (option == 1) { System.out.print("Enter name: "); // scanner takes in input } else if (option == 2) { System.out.print("Enter desc: "); // scanner takes in input } type = list.get((option - 1)); } while (option <= 0 || option >= 3);
However, when I entered anything larger than 2 for option, it threw me IndexOutOfBounds exception but I thought I did a try catch for it already?
Thanks in advance.
-
Admin about 8 yearsI figured out that the problem came from this line: type = list.get((option - 1));. After I surround it with a try catch, the error is gone but the problem is, when user entered anything larger than 2, it does not prompt for input again
-
Admin about 8 yearsHello, that's what I thought but it does not prompt user to enter input again thou. As in in I entered larger than 2, the first for loop that part wont print out again and ask for input
-
VinhNT about 8 yearsWhat do you got in console?
-
Admin about 8 yearsjust an empty space and I have to enter any value then the for loop that part will come out after that. Ah alright I knew already because of the sc.next() in last try catch and that 's why
-
VinhNT about 8 yearsYou need to put some promt into console, for example System.out.print("Re-enter the option: ");