How to redirect to a asp.net core razor page (no routes)
Solution 1
Try this in view;
@using (Html.BeginForm())
{
<input type="submit" id="Submit">
}
Solution 2
@Roman Pokrovskij This might be way too much old but if you want to redirect to an Area you should:
return RedirectToPage ( "/Page", new { Area = "AreaName" } );
Solution 3
check out the MS page https://docs.microsoft.com/en-us/aspnet/core/mvc/razor-pages/?tabs=visual-studio
The associations of URL paths to pages are determined by the page's location in the file system. The following table shows a Razor Page path and the matching URL:
File name path matching URL --------------------------- ---------------------- /Pages/Index.cshtml / or /Index /Pages/Contact.cshtml /Contact /Pages/Store/Contact.cshtml /Store/Contact /Pages/Store/Index.cshtml /Store or /Store/Index
URL generation for pages supports relative names. The following table shows which Index page is selected with different RedirectToPage parameters from Pages/Customers/Create.cshtml:
RedirectToPage(x) Page ------------------------ --------------------- RedirectToPage("/Index") Pages/Index RedirectToPage("./Index"); Pages/Customers/Index RedirectToPage("../Index") Pages/Index RedirectToPage("Index") Pages/Customers/Index
Solution 4
If you want to redirect to a different page after some action:
return Redirect("~/Page/YourAction");
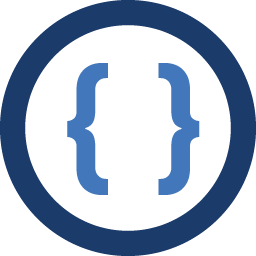
Admin
Updated on July 05, 2022Comments
-
Admin almost 2 years
Here I have a razor cs page:
public IActionResult OnPost(){ if (!ModelState.IsValid) { return Page(); } return RedirectToPage('Pages/index.cshtml'); }
And a cshtml page:
@page @using RazorPages @model IndexModel <form method="POST"> <input type="submit" id="Submit"> </form>
I want to redirect to the same page on form submit but I keep getting 400 error. Is it possible to do the redirect without using routes and just go to cshtml file in the url?
-
Admin over 6 yearsThis works when RedirectToPage('Pages/index.cshtml'); is changed to RedirectToPage('Index');
-
ssmith over 6 yearsYou could also just use RedirectToPage();
-
Roman Pokrovskij over 5 yearsHi, could I ask you how we should redirect to pages in the areas: /Areas/MyArea/Pages/Index.cshtml?
-
DeveloperDan over 4 yearsThe big GOTCHA for me was thinking I needed "Pages" or "/Pages" in the path. Once I removed it everything worked. REMOVE "Pages" FROM YOUR REDIRECT PATH.