how to refresh message stream for chat inbox in flutter
It's been a while since this question was originally asked. Since then the Stream Chat Flutter support has improved a lot. Implementing what this question asks is now really easy, with varying levels of customisability.
Pre-built UI widgets package : https://pub.dev/packages/stream_chat_flutter
- This package is plug and play to add chat support, with a lot of customisability options.
If you want more control: https://pub.dev/packages/stream_chat_flutter_core
- This package provides convenient builders to enable you to build your own UI components. It does the heavy lifting for you but will require more implementation on your side.
If you want low level control: https://pub.dev/packages/stream_chat
Check out the tutorial for an easy getting started guide: https://getstream.io/chat/flutter/tutorial/
See here for awesome Stream examples of what you can build: https://github.com/GetStream/flutter-samples
Video tutorials: https://www.youtube.com/playlist?list=PLNBhvhkAJG6t-BxkRAnSqa67lm5C1mpKk
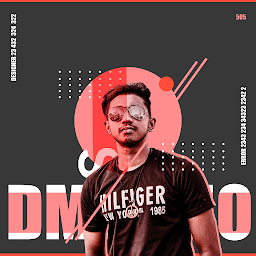
Dileepa Nipun Salinda
Designer (UI & UX) I am a designer who interested about great User Interface designing and User Experience Designing. Still studying to be a good UI designer.
Updated on December 16, 2022Comments
-
Dileepa Nipun Salinda over 1 year
I'm new to the flutter, I start to create a simple messenger app using flutter and flutter stream to handle API call for get message content.
also created the message controller to update and refresh the message list
class MessageService { Client httpClient = Client(); List<MessageModal> _messageList = []; Future<AppConfig> _getApiURL() async { final config = await AppConfig.forEnvironment('dev'); return config; } Future<List<MessageModal>> getMessageThread( String senderId, String receiverId) async { var config = await _getApiURL(); var url = config.baseUrl + "message/history"; final response = await httpClient.post(url, headers: {"content-type": "application/json"}, body: json.encode({ "senderId": senderId, "receiverId": receiverId, })); if (response.statusCode == 200) { _messageList = messageListFromJson(response.body); } else { _messageList = []; } return _messageList; } }
Here is the message service class for fetch API data
class MessageService { Client httpClient = Client(); List<MessageModal> _messageList = []; Future<AppConfig> _getApiURL() async { final config = await AppConfig.forEnvironment('dev'); return config; } Future<List<MessageModal>> getMessageThread( String senderId, String receiverId) async { var config = await _getApiURL(); var url = config.baseUrl + "message/history"; final response = await httpClient.post(url, headers: {"content-type": "application/json"}, body: json.encode({ "senderId": senderId, "receiverId": receiverId, })); if (response.statusCode == 200) { _messageList = messageListFromJson(response.body); } else { _messageList = []; } return _messageList; } }
Here is the ui preview to create the message list preview
StreamBuilder<List<MessageModal>> _buildStreamBuilder() { return StreamBuilder<List<MessageModal>>( // stream: _messageService.getMessageThread("UID1", "UID2").asStream(), stream: streamController.counter, initialData: _messageList, builder: (BuildContext context, AsyncSnapshot<List<MessageModal>> snapshot) { print(snapshot.data); if (snapshot.hasError) { print(snapshot.error); return Center( child: Text("Something went wrong!"), ); } else if (snapshot.hasData) { List<MessageModal> messages = snapshot.data; return _buildMessageHistory(messages); } else { return Center( child: CircularProgressIndicator(), ); } }, ); }
I need to do the messages update and also keep updating (send the API call and fetch data to stream) the message preview. can anybody help me on this one.