How to remove border from iframe in IE using javascript
Solution 1
Bizarrely, I was looking for an answer to this very issue myself earlier today. I found that setting the frameBorder
to 0
property does work, so long as you do it before the iframe is added to the document.
var iframe = document.createElement("iframe");
iframe.frameBorder = 0;
document.body.appendChild(iframe);
Solution 2
The frameBorder attribute exists directly on the iframe element, is not a CSS property.
Try with:
iframeElement.frameBorder = 0;
Solution 3
Try this. It will find any iframe elements and remove their borders in IE and other browsers (though you can just set a CSS style of "border : none;" in non-IE browsers instead of using JavaScript). AND it will work even if used AFTER the iframe is generated and in place in the document (e.g. iframes that are added in plain HTML and not JavaScript)!
This appears to work because IE creates the border, not on the iframe element as you'd expect, but on the CONTENT of the iframe--after the iframe is created in the BOM. ($@&*#@!!! IE!!!)
Note: The IE part will only work (of course) if the parent window and iframe are from the SAME origin (same domain, port, protocol etc.). Otherwise the script will get "access denied" errors in the IE error console. If that happens, your only option is to set it before it is generated, as others have noted, or use the non-standard frameBorder="0" attribute. (or just let IE look fugly--my current favorite option ;) )
Took me MANY hours of working to the point of despair to figure this out...
Enjoy. :)
// =========================================================================
// Remove borders on iFrames
if (window.document.getElementsByTagName("iframe"))
{
var iFrameElements = window.document.getElementsByTagName("iframe");
for (var i = 0; i < iFrameElements.length; i++)
{
iFrameElements[i].frameBorder="0"; // For other browsers.
iFrameElements[i].setAttribute("frameBorder", "0"); // For other browsers (just a backup for the above).
iFrameElements[i].contentWindow.document.body.style.border="none"; // For IE.
}
}
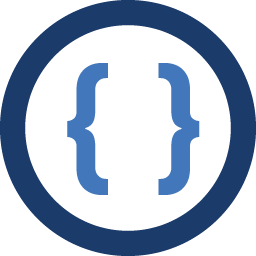
Admin
Updated on March 15, 2020Comments
-
Admin about 4 years
I am trying to insert an iframe into the browser DOM via javascript and want to remove the border if IE but can't seem to. I have tried these to no avail:
iframeElement.style.borderStyle="none";
and
iframeElement.style.frameBorder = "0";
Any suggestions will be much appreciated.