How to resize an image inside an UIButton programmatically?
Solution 1
This can be done through code in the following way:
let imageSize:CGSize = CGSize(width: 20, height: 20)
let button:UIButton = UIButton(type: UIButton.ButtonType.custom)
button.frame = CGRect(x: 200, y: 200, width: 60, height: 60)
button.backgroundColor = UIColor.yellow
button.setImage(UIImage(named: "chat.png"), for: UIControl.State.normal)
// The below line will give you what you want
button.imageEdgeInsets = UIEdgeInsets(
top: (button.frame.size.height - imageSize.height) / 2,
left: (button.frame.size.width - imageSize.width) / 2,
bottom: (button.frame.size.height - imageSize.height) / 2,
right: (button.frame.size.width - imageSize.width) / 2)
self.view.addSubview(button)
This way, you can achieve what you wanted.
Solution 2
You can experiment with image view insets. Every UIButton has a property imageView.
In Swift 3 you can do this like so:
//let button = UIButton()
button.imageView?.backgroundColor = UIColor.red
button.imageEdgeInsets = UIEdgeInsetsMake(10, 10, 10, 10)
red background is just so you know what is changing
Solution 3
I couldn't get the button's imageView to resize until I used contentHorizontalAlignment and contentVerticalAlignment both set to .fill. Then using imageEdgeInsets I repositioned the image.
let button = UIButton()
let image = UIImage(systemName: "bag.badge.plus")
button.setImage(image, for: .normal)
button.contentHorizontalAlignment = .fill
button.contentVerticalAlignment = .fill
button.imageEdgeInsets = UIEdgeInsets(top: 6, left: 6, bottom: 10, right: 10)
Result:
Solution 4
I would do it this way:
A UIButton
is just a UIView
. You can simply add a UIImageView
with a set image and call addSubview
on the UIButton
.
Solution 5
Taking into account what KVISH said before i have implemented this and it worked as expected. I posted this because Houman asked for an example.
//grab the image using the name of the pic
var image = UIImage(named: "picture")
//set the size for the image
image = image?.resize(toWidth: 18)
image = image?.resize(toHeight: 18)
//set the image to the button
buttonName.setImage(image, for: UIControlState.normal)
//adjust the position
buttonName.imageEdgeInsets = UIEdgeInsetsMake(8,16,9,0)
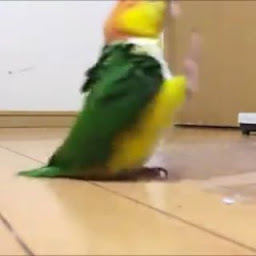
Elia Crocetta
Updated on July 09, 2022Comments
-
Elia Crocetta almost 2 years
I have this UIButton and an image to fit in. I don't want that the image take all the space inside the button but just a little part of it right in the center, but if I resize the button it will resize the image too. How can I do that, is there an option to set whatever dimension I want independently from the size of the UIButton? Thanks!