Check if Timer is running
10,626
Solution 1
I recommend to use self-checking startTimer()
and stopTimer()
functions, the timer will be started only if it's not currently running, the stop function sets the timer
variable reliably to nil
.
var timer : Timer?
func startTimer()
{
if timer == nil {
timer = Timer.scheduledTimer...
}
}
func stopTimer()
{
if timer != nil {
timer!.invalidate()
timer = nil
}
}
Solution 2
You need to check if your Timer
instance isValid
(not the Timer
class), let's say: if myTimer.isValid? {}
.
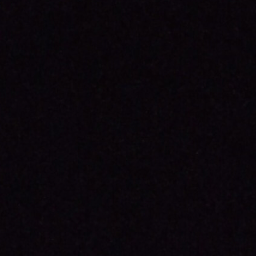
Author by
Jack Sullivan
Updated on June 15, 2022Comments
-
Jack Sullivan almost 2 years
I have been trying to refill in game lives with a timer, but whenever I leave a view and return, the timer duplicates and becomes faster. I have tried to address this with the
Timer?.isValid
function to only run the timer when it is invalid so it never duplicates, but it cannot seem to check is the timer is invalid in an if statement.This is the if statement that I have been using so far:
if (myTimer?.isValid){ } else{ //start timer }
Any help would be appreciated, thanks.
-
Jack Sullivan almost 7 yearsim not sure if you understand, i want to check if a timer is running, not start/stop a timer
-
vadian almost 7 yearsYour code in the question implies: If the timer is not valid (is not running), start it. That's exactly what
startTimer()
does. In my suggested logic the timer is not running if it'snil
. -
Jack Sullivan almost 7 yearsSorry, I understand now and your function is working!