How to retrieve all permissions assigned to a group in Django
Solution 1
The Group
model has a ManyToMany
relationship with the Permission
model, so you can do this
for group in Group.objects.all():
permissions = group.permissions.all()
# do something with the permissions
Solution 2
To get all permissions in all groups the current user belongs to use this:
all_permissions_in_groups = user.get_group_permissions()
To get all permissions related to a user including ones that are not in any group do this:
all_permissions = user.get_all_permissions()
For more details see Django documentation
Related videos on Youtube
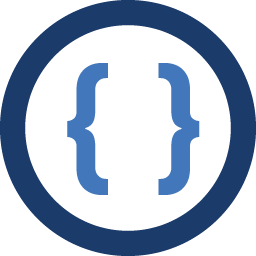
Admin
Updated on July 31, 2022Comments
-
Admin almost 2 years
I am working on a task to retrieve a set of permissions assigned to a group in Django and I can get the created groups using the below code, but could not use it to get the permissions assigned to them:
from django.contrib.auth.models import Group, Permission groups = Group.objects.all()
I am not really sure how to get permissions assigned to these groups.
permissions = Permission.objects.all()
gives me all the permissions, but I could not figure out how to get permissions assigned to a particular group.Any pointers would be appreciated.
-
Sardorbek Imomaliev over 9 yearsdo not do query in loop! in this case better to do something like this
group_ids = Group.objects.all().values_list('id', flat=True) Permission.objects.filter(group__id__in=group_ids)
this way u will always have only 2 queries. -
Manish Shah over 3 yearsHey @SardorbekImomaliev can you throw some light on this :- stackoverflow.com/questions/65156217/…