How to Return an Array of Strings in C?
Solution 1
There seem to be some confusion about what a string is in C. In C, a null terminated sequence of char
s is considered a string. It is usually represented by char*
.
I just want to call the
build_array()
function and return the array of strings
You pretty much can't return an array, neither a pointer to a local array. You could however pass the array to build_array
as an argument, as well as its size
, and fill that instead.
void build_array( char* strings[], size_t size )
{
// make sure size >= 2 here, based on your actual logic
strings[0] = "A";
strings[1] = "B";
}
...later called as:...
char *array[NUMBER];
build_array(array, NUMBER);
The alternatives are to return a pointer to a global or static allocated array, which would make your function non-reentrant. You probably don't care about this now, but is bad practice so I would recommend you avoid going that route.
Solution 2
As littleadv pointed out, there are several problems with your code:
Mismatch between
char **
andchar **[ ]
Returning a pointer to a local variable
Etc.
This example might help:
#include <stdio.h>
#include <string.h>
#include <malloc.h>
#define NUMBER 2
#define MAX_STRING 80
char **
build_array ()
{
int i = 0;
char **array = malloc (sizeof (char *) * NUMBER);
if (!array)
return NULL;
for (i = 0; i < NUMBER; i++) {
array[i] = malloc (MAX_STRING + 1);
if (!array[i]) {
free (array);
return NULL;
}
}
strncpy (array[0], "ABC", MAX_STRING);
strncpy (array[1], "123", MAX_STRING);
return array;
}
int
main (int argc, char *argv[])
{
char **my_array = build_array ();
if (!my_array) {
printf ("ERROR: Unable to allocate my_array!\n");
return 1;
}
else {
printf ("my_array[0]=%s, my_array[1]=%s.\n",
my_array[0], my_array[1]);
}
return 0;
}
Solution 3
Your return type is char**
, while you're assigning it to char**[]
, that's incompatible.
Other than that you should post the actual code that you have problem with, the code you posted doesn't compile and doesn't make much sense.
In order to fix your code, the function should be returning char **[NUMBER]
. Note also, that you're casting the return value to char*
instead of char**
that you declared (or char **[NUMBER]
that it should be, and in fact - is).
Oh, and returning a pointer to a local variable, as you do in your case, is a perfect recipe for crashes and undefined behavior.
What you probably meant was:
char *array[NUMBER];
int ret = build_array(array, NUMBER);
// do something with return value or ignore it
and in an imported file
int build_array(char **arr, int size)
{
// check that the size is large enough, and that the
// arr pointer is not null, use the return value to
// signal errors
arr[0] = "A";
arr[1] = "B";
return 0; // asume 0 is OK, use enums or defines for that
}
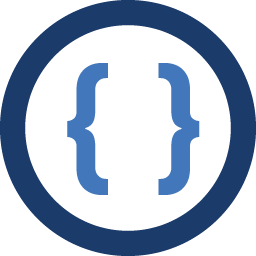
Admin
Updated on June 20, 2020Comments
-
Admin almost 4 years
For example, I have in the main file
1) char ** array[NUMBER]; 2) array = build_array();
and in an imported file
char ** build_array() { char ** array[NUMBER]; strings[0] = "A"; strings[1] = "B"; return (char *) strings; }
However, at line 2 in the main file, I get the error:
"incompatible types when assigning to type 'char **[(unsighed int)NUMBER]' from type 'char **'
What am I doing wrong? Any suggestions or advice would be appreciated. Thank you in advance.
-
Admin over 12 yearsWhat should the return type be to return an array of strings?
-
Admin over 12 yearsThank you so much. It now compiles, however, when I try to print the contents, nothing prints or gibberish prints. Is there an issue with assigning values to the array?
-
K-ballo over 12 years@LearningPython: We have seen so little of your code, that I wouldn't know. How is
NUMBER
defined? How do you attempt to print the contents? -
K-ballo over 12 years@LearningPython: Well I'm intrigued... what was it?
-
visual_learner over 12 yearsUsing
int
for an array size? Really? ;) -
K-ballo over 12 years@Chris Lutz: If in C++ I would have gone with
std::size_t
which is unsigned, is that your point? -
visual_learner over 12 years@K-ballo -
size_t
is a type in C as well, it's the type of the value returned bysizeof
. (size_t
is declared instddef.h
andstdlib.h
)