How to run Angular with https when by default it runs with http?
Solution 1
Simple fix is instead of running:
ng s -o
Run it with an extra attribute:
ng s -o ---ssl true
It will run on https://localhost:4200
. But, if you have a .crt
and a .key
file, then add that attribute also.
You will see a browser which will start with https, although it will say "not secure" as a warning.
This is sufficient if you just wanna run on https and you don't care about the "not secure" message. If you do care, proceed with further instructions.
ng s -o ---ssl true --ssl-key <path to key file> --ssl-cert <path to crt file>
or give relative path to .key
and .crt
file.
If you don't want to provide these attributes each time, or running for example a full NGINX server for angular, then add these attributes in angular.json
or angular-cli.json
depending upon angular version:
"serve":
{
"builder": "@angular-devkit/build-angular:dev-server",
"options":
{
"browserTarget": "ideationapp:build",
"ssl": true,
"sslKey": "ssl/server.key",
"sslCert": "ssl/server.crt",
}
}
sslkey
and sslcert
are not required if you don't have .key
and .crt
file.
Here, I assumed to have both files in a ssl
dir where src. Now, runningonly ng s -o
is sufficient to use the certificate via the angular.json
file.
How to create temporary fix for localhost for only your machine or only for one users.
Requirements:
- Git bash (Download fom here)
Now go to Git bash and type this command one at a time
git clone https://github.com/RubenVermeulen/generate-trusted-ssl-certificate.git(cloned to local computer)
cd generate-trusted-ssl-certificate(Going to application path)
bash generate.sh(starting shell script wher we called openssl)
The cloned application use openssl(software library for applications that secure communications over computer networks against eavesdropping or need to identify the party at the other end) to generate .crt and .key file
It will create server.key
and server.crt
file.
Now click on server.crt
For OS X
- Double click on the certificate (server.crt)
- Select your desired keychain (login should suffice)
- Add the certificate
- Open Keychain Access if it isn’t already open
- Select the keychain you chose earlier
- You should see the certificate localhost
- Double click on the certificate
- Expand Trust
- Select the option Always Trust in When using this certificate
- Close the certificate window
Windows 10
- Double click on the certificate (server.crt)
- Click on the button “Install Certificate …”
- Select whether you want to store it on user level or on machine level
- Click “Next”
- Select “Place all certificates in the following store”
- Click “Browse”
- Select “Trusted Root Certification Authorities”
- Click “Ok”
- Click “Next”
- Click “Finish”
- If you get a prompt, click “Yes”
The certificate is now installed.
Now store the certificate in a ssl
directory.
Now use the command line to provde ssl key and certificate, or add these files to angular.json
(or angular-cli.json
, depending on your Angular version).
You will not see any "not secure", and it will show "secure" if you click on lock icon adjacent to address bar.
But, if you will run the application in other's laptop it will show "not secure" as they have not installed the certificate (trusted).
Solution 2
To run angular application on https, do the following steps. npm install -g angular-http-server
Cd Path to site & in dist folder (ClientApp/dist)
Angular-http-server -o
By default runs at 8080 port and with http
Specify a port using -p e.g. Angular-http-server -p 44367 -o
To enable https, you have to manually specify the paths to your self-signed certificate using the --key and --cert flags
angular-http-server --https --key c:/localhost.key --cert c:/localhost.crt -p 44367 -o OR angular-http-server --https --key "Path where key stores" --cert "Path"
you probably see some error at this point if ssl not installed on local machine.
If OpenSSL is not installed on machine, download from below link
http://slproweb.com/download/Win64OpenSSL-1_1_0L.exe
Command to generate OpenSSL
openssl req -new -x509 -newkey rsa:2048 -sha256 -nodes -keyout localhost.key -days 3560 -out localhost.crt -config certificate.cnf
below link is describing how to create a certificate, configuring CLI to use
https and Trusting the certificate on Windows.
https://medium.com/@richardr39/using-angular-cli-to-serve-over-https-locally-70dab07417c8
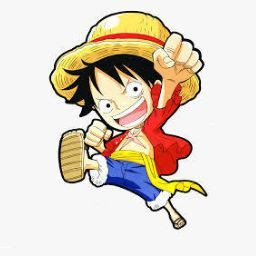
Comments
-
Satish Patro almost 2 years
By default if we run Angular applications they run with
http
(e.g. onhttp://localhost:4200
).How to convert it from
http
to usehttps
? -
saurabh kamble about 5 yearsupdate this answer in question dont worry about down vote @P Satish
-
Satish Patro about 5 yearsI don't understand how to add answer in question and this is the recommended way when you ask question, it will show a checkbox to answer your question, which I followed. Am I wrong or not appropriate way @saurabhkamble?
-
saurabh kamble about 5 yearsyou are correct dont worry about the down votes @P Satish
-
vimalDev over 4 yearswith https option getting error "Unknown option: '--https'"
-
Reza Kajbaf over 3 yearsI believe its supposed to be --ssl not --https