How to save an image as a variable?
Solution 1
Continuing @eatmeimadanish's thoughts, you can do it manually:
import base64
with open('image.gif', 'rb') as imagefile:
base64string = base64.b64encode(imagefile.read()).decode('ascii')
print(base64string) # print base64string to console
# Will look something like:
# iVBORw0KGgoAAAANS ... qQMAAAAASUVORK5CYII=
# or save it to a file
with open('testfile.txt', 'w') as outputfile:
outputfile.write(base64string)
# Then make a simple test program:
from tkinter import *
root = Tk()
# Paste the ascii representation into the program
photo = 'iVBORw0KGgoAAAANS ... qQMAAAAASUVORK5CYII='
img = PhotoImage(data=photo)
label = Label(root, image=img).pack()
This is with tkinter PhotoImage though, but I'm sure you can figure out how to make it work with PIL.
Solution 2
Here is how you can open it using PIL. You need a bytes representation of it, then PIL can open a file like object of it.
import base64
from PIL import Image
import io
with open("picture.png", "rb") as file:
img = base64.b64encode(file.read())
img = Image.open(io.BytesIO(img))
img.show()
Related videos on Youtube
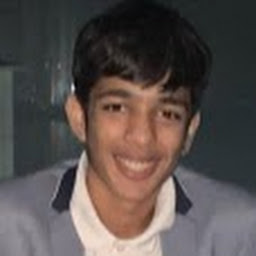
ѕняєє ѕιиgнι
I am 14 years old, I love programming. I know a C++ basics, and I am very fluent in Python ( my favourite programming language). I have learnt Javascript and HTML, but it only took my a few does to forget them. Currently looking for stuff in machine learning (it fascinates me)
Updated on June 04, 2022Comments
-
ѕняєє ѕιиgнι almost 2 years
Now, I have a python game that has sprites, and it obtains the images from files in its directory. I want to make it such that I do not even need the files. Somehow, to pre-store the image in a variable so that i can call it from within the program, without the help of the additional .gif files
The actual way i am using the image is
image = PIL.Image.open('image.gif')
So it would be helpful if you could be precise about how to replace this code
-
Santosh Kumar over 5 yearsDoesn't tkinter has an image object? Qt has one, named QImage.
-
ѕняєє ѕιиgнι over 5 yearsbut you have to open the image each time from the file
-
Aran-Fey over 5 yearsWhy? Why do you think it's a good idea to embed images in code?
-
roganjosh over 5 yearsAnd when the program ends, what happens to the image data?
-
ѕняєє ѕιиgнι over 5 yearsi want to convert the .py into .exe, and i want everything to bo neat. And i only want to carry one file around
-
ѕняєє ѕιиgнι over 5 years@rojanghosh The data should still be there so that i can access it again next time i run the code
-
Aran-Fey over 5 years"How do I package my program into an exe" is a much better question than "how do I put images into python scripts". And the answer definitely won't be "Put all the data in your python script".
-
Aran-Fey over 5 years
-
ѕняєє ѕιиgнι over 5 yearsI know how to convert it into an executable, but I did not know how to save the image in the code, so that the executable can handle it
-
Russell Smith over 5 years@Aran-Fey: I think you're being a bit harsh. I think it's perfectly reasonable to embed some image data in a script under some circumstances. It may not be a best practice, but it's still a reasonable solution.
-
Aran-Fey over 5 years@BryanOakley Hmm, I think I can see what you mean. But that really wasn't intentional. I smelled an XY problem, so I asked a question to find out if I was right, and then I simply stated that it would've been better to ask about the X rather than the Y. I can see why you would think that I was... hmmm... cold, but it's really all factual and neutral.
-
-
ѕняєє ѕιиgнι over 5 yearsWhat would the value of
img
be an alias for? -
ѕняєє ѕιиgнι over 5 yearsCould you please specify how to implement this in PIL, I am having difficulties
-
figbeam over 5 yearsI don't use PIL an haven't installed it, so I can not give you advice on this other than to suggest you look at: How to convert base64 string to a PIL Image object.
-
U12-Forward over 5 yearsWhere's your explanation?
-
eatmeimadanish over 5 yearsWe got there at the end.