how to save base64 image decode to public folder in laravel
Solution 1
//Controller
use Illuminate\Support\Facades\Storage;
//Inside method
$image = $request->image; // your base64 encoded
$image = str_replace('data:image/png;base64,', '', $image);
$image = str_replace(' ', '+', $image);
$imageName = str_random(10) . '.png';
Storage::disk('local')->put($imageName, base64_decode($image));
Also, make sure that your local
disk is configured like that in /config/filesystems.php
'local' => [
'driver' => 'local',
'root' => storage_path('app/public'),
]
With that, file will be saved in /storage/app/public
directory.
Don't forget to write php artisan storage:link
to make files from that directory available in /public
directory, so users will can retrieve them.
Solution 2
Saving image in database not a recommanded way to store image in db, reason is speed increase of memory, The correct way is just save the link to it.
Anyway, you can save your image in base64
with these line of code.
Get the correct image from path in your server not from link in the $path
.
<?php
// Base 64 transform image
$path = __DIR__ .'/myfolder/myimage.png';
$type = pathinfo($path, PATHINFO_EXTENSION);
$data = file_get_contents($path);
$base64 = 'data:image/' . $type . ';base64,' . base64_encode($data);
echo $base64;
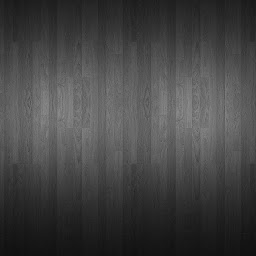
Rahmat Effendi
Updated on June 11, 2022Comments
-
Rahmat Effendi almost 2 years
I get an image with format String base64 and i want to decode that string to be an image and save it to public folder in laravel.
This is my controller:
//decode string base64 image to image $image = base64_decode($request->input('ttd')); //create image name $photo_name = time().'.png'; $destinationPath = public_path('/uploads'); //save image to folder $image->move($destinationPath, $photo_name); $img_url = asset('/uploads'.$photo_name); $data = new Transaction(); $data->transaction_id = $request->input('fa_transaction_id'); $data->user_id = $request->input('userid'); $data->photo_name = $photo_name; $data->photo_url = $img_url; $data->save();
when i'm trying echo $image, i got the decode value, and also for $photo_name i got the value too, but when the function running i got this error
Call to a member function move() on string
how to fix this error?