How to select multiple items from a List view - JavaFX 8
11,492
Here's an example based on your comments
import javafx.application.Application;
import javafx.collections.FXCollections;
import javafx.collections.ObservableList;
import javafx.scene.Scene;
import javafx.scene.control.ListView;
import javafx.scene.control.SelectionMode;
import javafx.scene.layout.HBox;
import javafx.stage.Stage;
public class ListSelect extends Application {
@Override
public void start(Stage stage) {
ObservableList<String> items = FXCollections.observableArrayList(
"one","two","three","four","five","six","seven");
ListView<String> list = new ListView<>(items);
ListView<String> selected = new ListView<>();
HBox root = new HBox(list, selected);
Scene scene = new Scene(root);
stage.setScene(scene);
stage.show();
//set this to SINGLE to allow selecting just one item
list.getSelectionModel().setSelectionMode(SelectionMode.MULTIPLE);
list.getSelectionModel().selectedItemProperty().addListener((obs,ov,nv)->{
selected.setItems(list.getSelectionModel().getSelectedItems());
});
}
public static void main(String[] args) {launch(args);}
}
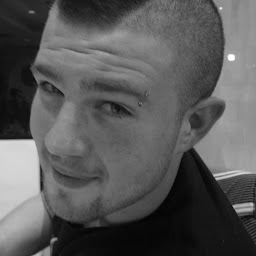
Author by
Gurman Eduard
Updated on June 16, 2022Comments
-
Gurman Eduard almost 2 years
I'm new at using
JavaFX
and I'm trying to add anObservableList
to a table view. The list contains only String.My goals is to show list of connected devices and let the user choose on which to perform the action (1 or more), is there any better way to achieve this?
Edit: Ive chaned to ListView and now it shows the list, how can I create a new list from the selected Items ?