How to send an array in dart post request
8,241
Solution 1
Problem solved; I just encoded the body:
List<String> tokens=["token1","token2"];
final url='https://fcm.googleapis.com/fcm/send';
http.post(url,headers:{
"Accept": "application/json",
"Authorization":"key=mykey"
,"project_id":"proID"
},
body:json.encode(
{
"registration_ids" :tokens ,
"collapse_key" : "type_a",
"notification" : {
"body" : "Body of Your Notification",
"title": "Title of Your Notification"
}
)
}
Solution 2
you are having problems due to the registration is expecting a JSON
string but you are passing list object to it. You can simply resolve that by casting your List<String>
to String
.
By casting the token list using toString()
method you will get a String like this "['token1','token2']"
Here's the modified code:
List<String> tokens=["token1","token2"];
final url='https://fcm.googleapis.com/fcm/send';
http.post(url,headers:{
"Accept": "application/json",
"Authorization":"key=mykey"
,"project_id":"proID"
},
body:
{
"registration_ids" : tokens.toString() ,
"collapse_key" : "type_a",
"notification" : {
"body" : "Body of Your Notification",
"title": "Title of Your Notification"
}
}
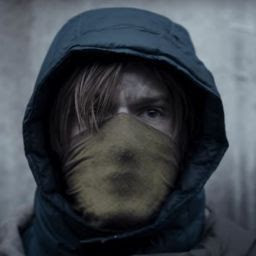
Author by
karrar jasim
Updated on November 19, 2022Comments
-
karrar jasim over 1 year
I try to send messages to device groups with their
registration_ids
.This my code:
List<String> tokens=["token1","token2"]; final url='https://fcm.googleapis.com/fcm/send'; http.post(url,headers:{ "Accept": "application/json", "Authorization":"key=mykey" ,"project_id":"proID" }, body: { "registration_ids" :tokens , "collapse_key" : "type_a", "notification" : { "body" : "Body of Your Notification", "title": "Title of Your Notification" } }
When the app runs this error displays:
Exception has occurred. _CastError (type 'List' is not a subtype of type 'String' in type cast)
How to fix it?
-
Haley Huynh almost 3 yearsnot working, it is passing just as string not a list.