How to send binary data via JQuery Ajax PUT method
Most (all?) browsers will automatically convert string datatypes to UTF-8 when sending them via an XMLHttpRequest
. When you read the file as a binary string, you're given exactly that: a JavaScript string object where each character is a value between 0 and 255.
To force the AJAX request to send binary encoded data, the data parameter must be an ArrayBuffer
, File
, or Blob
object.
If you need access to the contents of the file before sending, you can convert the binary string to an ArrayBuffer
with the following code:
var arrBuff = new ArrayBuffer(file.result.length);
var writer = new Uint8Array(arrBuff);
for (var i = 0, len = file.result.length; i < len; i++) {
writer[i] = file.result.charCodeAt(i);
}
And you'd pass the arrBuff
instance to the AJAX function. Otherwise, you should be able to pass the File
object itself into the AJAX function without all of your FileReader
code.
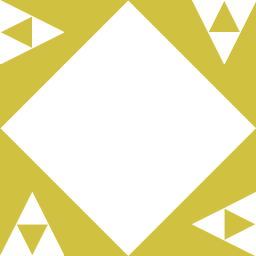
Ding
Updated on June 21, 2022Comments
-
Ding 7 months
I am new to JQuery and I want to use JQuery Ajax to upload some files to server, Only in PUT method. when I send some binary file(such as gif or jpeg) to my server, upload succeed, but the content of binary data was changed(it's always bigger than original file size). I try to change the content-type or the type of file result, but still not work. Anyone knows how to fix this?
PS: I cannot encode the binary file's content into other form, because I cannot touch the code of server.
var reader = new FileReader(); reader.onloadend = (function(Thefile) { $.ajax({ url: url, processData:false, //contentType:"application/octet-stream; charset=UTF-8", data: file.result, type: 'PUT', success : function(){console.log("OK!");}, error : function(){console.log("Not OK!");} }); })(file); reader.readAsBinaryString(file);