How to send push notifications in Flutter android app?
The simplest way is to use Firebase Cloud Messaging. Especially since Google is deprecating GCM which was previously used for Android. Also Firebase cloud messaging is free and can be used for both iOS and Android. Apple's APN service will require a setup as well though and a paid developer account.
Create a Firebase project if you haven't already and enable cloud messaging.
To set up your Node.js server so that it can send push notifications to your android and IOS devices. Click on the Project Overview, Settings and service accounts and follow the directions to generate a private key for your project and follow the instructions for setup. Also npm install "firebase-admin".
Once you have firebase setup refer to these docs for how to send messages. https://firebase.google.com/docs/cloud-messaging/send-message
There are several ways to send messages. You can send messages directly.
with this code
// This registration token comes from the client FCM SDKs.
var registrationToken = 'YOUR_REGISTRATION_TOKEN';
var message = {
data: {
score: '850',
time: '2:45'
},
token: registrationToken
};
// Send a message to the device corresponding to the provided
// registration token.
admin.messaging().send(message)
.then((response) => {
// Response is a message ID string.
console.log('Successfully sent message:', response);
})
.catch((error) => {
console.log('Error sending message:', error);
});
You can also create topics for devices to subscribe to as well if you are sending out mass notifications. More examples once again are within the docs. Now if you are wondering what the token is that is the next step.
- The token comes from the unique device that is connecting to your platform. You can get the token by installing the Firebase Messaging sdk from https://pub.dev/packages/firebase_messaging and following the instructions to add the necessary dependencies to your pubsec.yaml and properly configure your Android manifest and iOS files for the changes.
This package will give you methods to grab your communicate and to receive the notifications that you have sent form your Node.JS server.
Here is an example of grabbing the token from your device on the frontend in flutter.
final FirebaseMessaging _firebaseMessaging = FirebaseMessaging();
bool _initialized = false;
Future<void> init() async {
if (!_initialized) {
// For iOS request permission first.
_firebaseMessaging.requestNotificationPermissions();
_firebaseMessaging.configure(onMessage: (Map<String, dynamic> `enter code here`message) {
print('onMessage: $message');
Platform.isAndroid
? showNotification(message['notification'])
: showNotification(message['aps']['alert']);
return;
}, onResume: (Map<String, dynamic> message) {
print('onResume: $message');
return;
}, onLaunch: (Map<String, dynamic> message) {
print('onLaunch: $message');
return;
});
// For testing purposes print the Firebase Messaging token
String token = await _firebaseMessaging.getToken();
print("FirebaseMessaging token: $token");
_initialized = true;
}
}
At this point you would most likely save the token to your MongoDB database and associate the token with your user and that specific device. Of course you would have to also install the firebase core and for Flutter as well and do all of the necessary configurations.
You are still able to maintain your NodeJS API and MongoDB database and use free cloud messaging service to push your notifications for your server to your device.
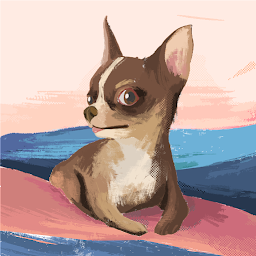
manoj kasun
Updated on December 19, 2022Comments
-
manoj kasun over 1 year
I developed a flutter android app. and my database is MongoDB. I use Node.js API to connect my flutter app with the MongoDB. I want to send push notifications when a new data record is coming to MongoDB. How can I do that?