How to set a timeout on a request with Apollo-Client
The Apollo client uses (v1.9 and apollo-link-http) the fetch API to send the requests. Therefore there is no cross-browser way to abort a fetch. You could create you own Networkinterface or Link and use something like this:
const oldfetch = fetch;
fetch = function(input, opts) {
return new Promise((resolve, reject) => {
setTimeout(reject, opts.timeout);
oldfetch(input, opts).then(resolve, reject);
});
}
But be careful as this doesn't actually abort the request. You can end up with a lot running requests and hit a browser limit.
BTW it looks like apollo-link-http is prepared for the AbortController ;-)
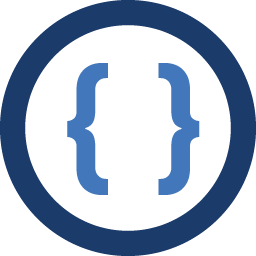
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
I have a React-Native app where I make requests to a GraphQL server. Everything works fine except I need a way to set the timeout on the request/client to 5 or 10 seconds. Currently the request takes a very long time before it times out (around 1 minute).
Here's a quick example of how I use the client.
const client = new ApolloClient({ networkInterface: createNetworkInterface({ uri: `${config.server_hostname}/graphql` }) }); client.query({ query: gqlString });
I have been unable to find any solution through StackOverflow, google search or Apollo's documentation.
-
David Harkness over 4 yearsAny idea if
AbortController
is operational yet? -
Johann Baptista over 4 yearsMozilla docs say it has full or partial support on most browsers now, but I'm thinking of using a polyfill just in case (npmjs.com/package/abortcontroller-polyfill), to impement the timeout.