How to set custom HTTP headers to requests made by a WKWebView
Solution 1
I've got it working in a way, but only get requests will have the custom header. As jbelkins answered in the linked so from Gabriel Cartiers comment to your question, you will have to manipulate the request and load it anew.
I've got it working for GET-Requests like this:
(it's in xamarin 0> c#, but i think you will get the idea)
i've created a private field
private bool _headerIsSet
which i check every time a request is made in the deligate method:
[Foundation.Export("webView:decidePolicyForNavigationAction:decisionHandler:")]
public void DecidePolicy(WKWebView webView, WKNavigationAction navigationAction, Action<WKNavigationActionPolicy> decisionHandler)
{
var request = navigationAction.Request;
// check if the header is set and if not, create a muteable copy of the original request
if (!_headerIsSet && request is NSMuteableUrlRequest muteableRequest);
{
// define your custom header name and value
var keys = new object[] {headerKeyString};
var values = new object[] {headerValueString};
var headerDict = NSDictionary.FromObjectsAndKeys(values, keys);
// set the headers of the new request to the created dict
muteableRequest.Headers = headerDict;
_headerIsSet = true;
// attempt to load the newly created request
webView.LoadRequest(muteableRequest);
// abort the old one
decisionHandler(WKNavigationActionPolicy.Cancel);
// exit this whole method
return;
}
else
{
_headerIsSet = false;
decisionHandler(WKNavigationActionPolicy.Allow);
}
}
As i said, this only works for GET-Requests. Somehow, POST-Requests don't contain the body data of the original request (request.Body and request.BodyStream are null), so the muteableRequest (which is a muteable copy of the original request) won't contain the body data of the original request.
I hope this will help you or others that approach the same problem.
Edit: For your needs, set "Accept-Language" as the key
Solution 2
Simply can set needed language ISO 639-1 code in URL request like below, so that we can get user preferred or locale language response from server side.
Swift 4 & above
var request = URLRequest(url: URL(string: "YourUrlStr"))
request.setValue("en", forHTTPHeaderField: "Accept-Language")
wkWebView.load(request)
Objective-C
NSMutableURLRequest *request = [NSMutableURLRequest requestWithURL:[NSURL URLWithString:YourUrlStr]];
[request setValue:@"en" forHTTPHeaderField:@"Accept-Language"];
[wkWebView loadRequest:urlRequest];
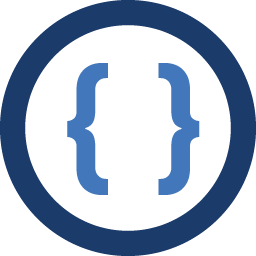
Admin
Updated on June 13, 2022Comments
-
Admin almost 2 years
I have built an app that includes a WKWebView, and the website that the web view loads supports multiple languages. How can I change the
Accept-Language
header in a WKWebView, or other HTTP headers for that matter? -
Alex Cohn over 2 yearsThis workaround has another problem except being limited to
GET
. It also assumes that the request it will intercept, replaces the whole content of the WebView. This isn't always true: if such secondary request is intended to change some elements of the page, e.g. an image displayed there, the result will be far from what was intended. -
DerDingens over 2 years@AlexCohn I'm not sure about that, did you encounter such a behavior? DecidePolicy should only be called if a navigation action is happening, e.g. the page changes
-
Alex Cohn over 2 yearsThis is exactly the problem: ajax requests, image requests, etc. are not handled by this approach.
-
DerDingens over 2 yearsOh okay, misunderstood your point there, true that won't be handled this way
-
Alex Cohn over 2 yearsThis can be achieved by intercepting the HTTP requests, but this may be like using a cannon to kill a fly.