How to set environment variables using Google Cloud Build or other method in Google App Engine Standard Environment?
Solution 1
Here is a tutorial on how to securely store env vars in your cloud build (triggers) settings and import them into your app.
Basically there are three steps:
-
Add your env vars to the 'variables' section in one of your build trigger settings
Screenshot of where to add variables in build triggers
By convention variables set in the build trigger must begin with an underscore (_)
-
Configure
cloudbuild.yaml
(on the second step in the code example) to read in variables from your build trigger, set them as env vars, and write all env vars in a local .env fileAdd
couldbuild.yaml
(below) to your project root directory
steps:
- name: node:10.15.1
entrypoint: npm
args: ["install"]
- name: node:10.15.1
entrypoint: npm
args: ["run", "create-env"]
env:
- 'MY_SECRET_KEY=${_MY_SECRET_KEY}'
- name: "gcr.io/cloud-builders/gcloud"
args: ["app", "deploy"]
timeout: "1600s"
Add create-env
script to package.json
"scripts": {
"create-env": "printenv > .env"
},
-
Read env vars from .env to your app (config.js)
Install dotenv package
npm i dotenv -S
Add a
config.js
to your app
// Import all env vars from .env file
require('dotenv').config()
export const MY_SECRET_KEY = process.env.MY_SECRET_KEY
console.log(MY_SECRET_KEY) // => Hello
Done! Now you may deploy your app by triggering the cloud build and your app will have access to the env vars.
Solution 2
I have another solution, if someone is still interested in this. This should work on all languages, because environment variables are added directly into app.yaml
file
Add substitution variable in build trigger (as described in this answer).
Add environment variables to
app.yaml
in a way they can be easily substituted with build trigger variables. Like this:
env_variables:
SECRET_KEY: %SECRET_KEY%
- Add a step in
cloudbuild.yaml
to substitute all%XXX%
variables insideapp.yaml
with their values from build trigger.
- name: 'gcr.io/cloud-builders/gcloud'
entrypoint: bash
args:
- '-c'
- |
sed -i 's/%SECRET_KEY%/'${_SECRET_KEY}'/g' app.yaml
gcloud app deploy app.yaml
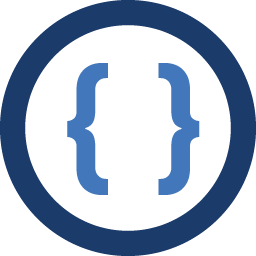
Admin
Updated on June 25, 2022Comments
-
Admin almost 2 years
Is there anyway to inject environment variables from Cloud Build into the App Engine Standard environment?
I do not want to push my environment variables to GitHub inside the
app.yaml
or.env
. Thus, when Cloud Build pulls and deploys it is missing the.env
file and the server is unable to complete some requests.I am trying to avoid using Datastore as the async nature of Datastore will make the code a lot more messy. I tried to use encrypted secrets found here, but that doesn't seem to work as I added the secrets to app deploy and they do not make their way into the deployment, so I assume this is not the use case for Cloud Build.
I also tried the tutorial here, to import the
.env
file into App Engine Standard from storage, but since Standard does not have local storage I assume it goes into the void.So is there anyway to inject the
.env
into App Engine Standard environment without using Datastore, or committingapp.yaml
or.env
to change control? Potentially using Cloud Build, KMS, or some type of storage?Here is what I tried for
cloudbuild.yaml
:steps: - name: "gcr.io/cloud-builders/gcloud" args: ["app", "deploy"] secretEnv: ['SECRET1', 'SECRET2', 'SECRET3', 'SECRET4', 'SECRET5'] timeout: "1600s" secrets: - kmsKeyName: projects/<Project-Name>/locations/global/keyRings/<Key-Ring-Name>/cryptoKeys/<Key-Name> secretEnv: SECRET1: <encrypted-key-base64 here> SECRET2: <encrypted-key-base64 here> SECRET3: <encrypted-key-base64 here> SECRET4: <encrypted-key-base64 here> SECRET5: <encrypted-key-base64 here>