How to sort List<Integer>?
Solution 1
You can use Collections
for to sort data:
import java.util.Collections;
import java.util.ArrayList;
import java.util.List;
public class tes
{
public static void main(String args[])
{
List<Integer> lList = new ArrayList<Integer>();
lList.add(4);
lList.add(1);
lList.add(7);
lList.add(2);
lList.add(9);
lList.add(1);
lList.add(5);
Collections.sort(lList);
for(int i=0; i<lList.size();i++ )
{
System.out.println(lList.get(i));
}
}
}
Solution 2
Ascending order:
Collections.sort(lList);
Descending order:
Collections.sort(lList, Collections.reverseOrder());
Solution 3
Use Collections class API to sort.
Collections.sort(list);
Solution 4
Just use Collections.sort(yourListHere)
here to sort.
You can read more about Collections from here.
Solution 5
You are using Lists, concrete ArrayList. ArrayList also implements Collection interface. Collection interface has sort method which is used to sort the elements present in the specified list of Collection in ascending order. This will be the quickest and possibly the best way for your case.
Sorting a list in ascending order can be performed as default operation on this way:
Collections.sort(list);
Sorting a list in descending order can be performed on this way:
Collections.reverse(list);
According to these facts, your solution has to be written like this:
public class tes
{
public static void main(String args[])
{
List<Integer> lList = new ArrayList<Integer>();
lList.add(4);
lList.add(1);
lList.add(7);
lList.add(2);
lList.add(9);
lList.add(1);
lList.add(5);
Collections.sort(lList);
for(int i=0; i<lList.size();i++ )
{
System.out.println(lList.get(i));
}
}
}
More about Collections you can read here.
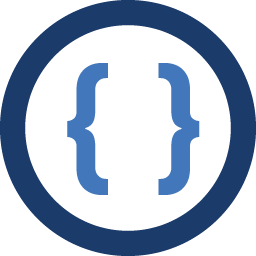
Admin
Updated on April 27, 2020Comments
-
Admin about 4 years
I have code and I have used list to store data. I want to sort data of it, then is there any way to sort data or shall I have to sort it manually by comparing all data?
import java.util.ArrayList; import java.util.List; public class tes { public static void main(String args[]) { List<Integer> lList = new ArrayList<Integer>(); lList.add(4); lList.add(1); lList.add(7); lList.add(2); lList.add(9); lList.add(1); lList.add(5); for(int i=0; i<lList.size();i++ ) { System.out.println(lList.get(i)); } } }