Java Sort List of Lists
28,991
Solution 1
You will have to implement your own Comparator
class and pass in an instance to Collections.sort()
class ListComparator<T extends Comparable<T>> implements Comparator<List<T>> {
@Override
public int compare(List<T> o1, List<T> o2) {
for (int i = 0; i < Math.min(o1.size(), o2.size()); i++) {
int c = o1.get(i).compareTo(o2.get(i));
if (c != 0) {
return c;
}
}
return Integer.compare(o1.size(), o2.size());
}
}
Then sorting is easy
List<List<Integer>> listOfLists = ...;
Collections.sort(listOfLists, new ListComparator<>());
Solution 2
Improved MartinS answer using Java 8 stream API
possiblePoles = possiblePoles.stream().sorted((o1,o2) -> {
for (int i = 0; i < Math.min(o1.size(), o2.size()); i++) {
int c = o1.get(i).compareTo(o2.get(i));
if (c != 0) {
return c;
}
}
return Integer.compare(o1.size(), o2.size());
}).collect(Collectors.toList());
Solution 3
For this example [[1, 3], [1, 2]], if you want to sort the list by both elements you can use sorted from Java 8 manually implemented the comparator method, validating all cases like the following example:
List<List<Integer>> result = contests.stream().sorted((o1, o2) -> {
if (o1.get(1) > o2.get(1) ||
(o1.get(1).equals(o2.get(1)) && o1.get(0) > o2.get(0))) {
return -1;
} else if (o1.get(1) < o2.get(1) ||
(o1.get(1).equals(o2.get(1)) && o1.get(0) < o2.get(0))) {
return 1;
}
return 0;
}).collect(Collectors.toList());
OR
contests.sort((o1, o2) -> {
if (o1.get(1) > o2.get(1) ||
(o1.get(1).equals(o2.get(1)) && o1.get(0) > o2.get(0))) {
return -1;
} else if (o1.get(1) < o2.get(1) ||
(o1.get(1).equals(o2.get(1)) && o1.get(0) < o2.get(0))) {
return 1;
}
return 0;
});
Related videos on Youtube
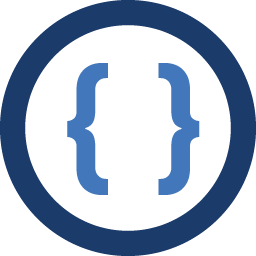
Author by
Admin
Updated on October 21, 2021Comments
-
Admin over 2 years
How would I sort a list of lists in Java in lexicographical order using Collections.sort() or another sorting method?
private List<List<Integer>> possiblePoles = setPoles(); System.out.println(possiblePoles) [[1, 3, 5], [1, 2, 3]]
-
MikeCAT about 8 yearsBy implementing sort by yourself?
-
Admin about 8 yearsNo, could be a Java function.
-
developer033 about 8 years
-
-
Nayuki about 8 yearsEven better: Because Java uses type erasure, you can make a singleton instance of ListComparator and do unsafe casts (it's stateless anyway).
-
MartinS about 8 years@Nayuki, I'd rather not do that because I do not want to carry around the instance forever, just because I used it once. The memory footprint might be tiny but so is the cost of creating an object. But everyone can do whatever they want ^^
-
Michael Anderson almost 7 yearsI'd use
int c = ObjectUtils.compare(o1.get(i), o2.get(i))
. Otherwise you get a NPE ifo1.get(i)==null
. (ObjectUtils
is from the apache commons library) -
Ole V.V. about 4 yearsSpecial case: If the lists are long linked lists, accessing by index is inefficient. In this case one should prefer to use two iterators.