How to split string in C without using strtok
Solution 1
- start with a pointer to the begining of the string
- iterate character by character, looking for your delimiter
- each time you find one, you have a string from the last position of the length in difference - do what you want with that
- set the new start position to the delimiter + 1, and the go to step 2.
Do all these while there are characters remaining in the string...
Solution 2
I needed to do this because the environment was working in had a restricted library that lacked strtok
. Here's how I broke up a hyphen-delimited string:
b = grub_strchr(a,'-');
if (!b)
<handle error>
else
*b++ = 0;
c = grub_strchr(b,'-');
if (!c)
<handle error>
else
*c++ = 0;
Here, a
begins life as the compound string "A-B-C"
, after the code executes, there are three null-terminated strings, a
, b
, and c
which have the values "A"
, "B"
and "C"
. The <handle error>
is a place-holder for code to react to missing delimiters.
Note that, like strtok
, the original string is modified by replacing the delimiters with NULLs.
Solution 3
This breaks a string at newlines and trims whitespace for the reported strings. It does not modify the string like strtok does, which means this can be used on a const char*
of unknown origin while strtok cannot. The difference is begin
/end
are pointers to the original string chars, so aren't null terminated strings like strtok gives. Of course this uses a static local so isn't thread safe.
#include <stdio.h> // for printf
#include <stdbool.h> // for bool
#include <ctype.h> // for isspace
static bool readLine (const char* data, const char** beginPtr, const char** endPtr) {
static const char* nextStart;
if (data) {
nextStart = data;
return true;
}
if (*nextStart == '\0') return false;
*beginPtr = nextStart;
// Find next delimiter.
do {
nextStart++;
} while (*nextStart != '\0' && *nextStart != '\n');
// Trim whitespace.
*endPtr = nextStart - 1;
while (isspace(**beginPtr) && *beginPtr < *endPtr)
(*beginPtr)++;
while (isspace(**endPtr) && *endPtr >= *beginPtr)
(*endPtr)--;
(*endPtr)++;
return true;
}
int main (void) {
const char* data = " meow ! \n \r\t \n\n meow ? ";
const char* begin;
const char* end;
readLine(data, 0, 0);
while (readLine(0, &begin, &end)) {
printf("'%.*s'\n", end - begin, begin);
}
return 0;
}
Output:
'meow !'
''
''
'meow ?'
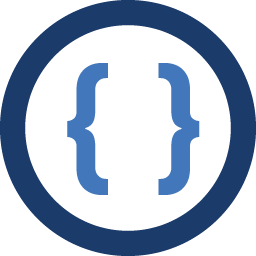
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
#include <stdio.h> int main() { char string[] = "my name is geany"; int length = sizeof(string)/sizeof(char); printf("%i", length); int i; for ( i = 0; i<length; i++ ) { } return 0; }
if i want to print "my" "name" "is" and "geany" separate then what do I do. I was thinking to use a delimnator but i dont know how to do it in C