How to store a string from a text file in an array in C
Solution 1
You only allocate one string array str
. At each iteration through the loop you are simply overwriting str
. The assignment x[i] = str
assigns the pointer value of str
to x[i]
. You'll notice that each member of the array x
points to the same buffer str
at the end of the loop. You need to create multiple buffers.
One way to do this is to define the maximum number of lines using #define LINES 100
and then to declare x
as follows
char x[LINES][MAX];
and then perform a strcpy
at each iteration:
while(fgets(str, sizeof str, fp)) {
strcpy(x[i], str);
printf("%s", str);
printf("%s", *(x+i));
i++;
}
Note that you should consider using the strncpy
method instead of strcpy
and check the return value to ensure that the buffers do not overrun.
Solution 2
You need to make a copy of each string:
x[i] = strdup(str);
What you're doing at the moment is making each x[i]
point to the same buffer, which will contain the last line of the file once you've finished.
(Note: you'll also need to free()
all of the x[i]
strings you create with strdup()
.)
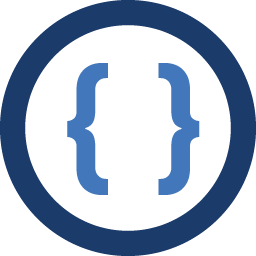
Admin
Updated on June 05, 2022Comments
-
Admin almost 2 years
I want to read a text file into a string array and be able to access the array contents through a loop. The code I have allows me to store only the last line of the text file instead of the entire file; where am I going wrong?
#define MAX 10000 int main (int argc, char *argv[]) { FILE *fp; char str[MAX]; char *x[MAX]; int i =0; char y[MAX]; if((fp = fopen("550.txt", "r"))==NULL) { printf("Cannot open file.\n"); exit(1);} while(!feof(fp)) { while(fgets(str, sizeof str, fp)) { x[i]= str; printf("%s", str); printf("%s", *(x+i)); i++; } } for(i=0;i<100;i++){ printf("%s", *(x+i)); } fclose(fp); return 0; }
-
Nyan over 13 yearsAre you sure that fgets will not zero-terminate the buffer for long input?