How to subset from a list in R
Solution 1
You could use sapply(mylist, "[", y)
:
mylist <- list(1:5, 6:10, 11:15)
sapply(mylist, "[", c(2,3))
Solution 2
Try using [
instead of [[
(and depending on what you're after you light actually want lapply
).
From ?'[['
:
The most important distinction between [, [[ and $ is that the [ can select more than one element whereas the other two select a single element.
Solution 3
Using lapply
:
# create mylist
list1<-1:10
list2<-letters[1:26]
list3<-25:32
mylist<-list(list1,list2,list3)
# select 3,5,9th element from each list
list.2 <- lapply(mylist, function(x) {x[c(3,5,9)]})
Solution 4
purrr provides another solution for solving these kinds of list manipulations within the tidyverse
library(purrr)
library(dplyr)
desired_values <- c(1,3)
mylist <- list(1:5, letters[1:6], 11:15) %>%
purrr::map(`[`,desired_values)
mylist
Solution 5
An easy way to subset repeated named elements of a list, similar to other answers here.
(so I can find it next time I look this question up)
E.g., subset the "b"
elements from a repeating list where each element includes an "a"
and "b"
sub-element:
mylist <- list(
list(
"a" = runif(3),
"b" = runif(1)
),
list(
"a" = runif(3),
"b" = runif(1)
)
)
mylist
#> [[1]]
#> [[1]]$a
#> [1] 0.7547490 0.6528348 0.2339767
#>
#> [[1]]$b
#> [1] 0.8815888
#>
#>
#> [[2]]
#> [[2]]$a
#> [1] 0.51352909 0.09637425 0.99291650
#>
#> [[2]]$b
#> [1] 0.8407162
blist <- lapply(
X = mylist,
FUN = function(x){x[["b"]]}
)
blist
#> [[1]]
#> [1] 0.8815888
#>
#> [[2]]
#> [1] 0.8407162
Created on 2019-11-06 by the reprex package (v0.3.0)
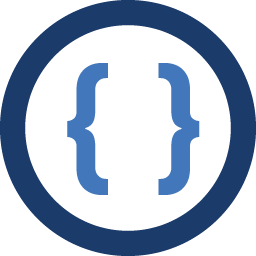
Admin
Updated on July 20, 2022Comments
-
Admin almost 2 years
I have a rather simple task but haven't find a good solution.
> mylist [[1]] [1] 1 2 3 4 5 6 7 8 9 10 [[2]] [1] "a" "b" "c" "d" "e" "f" "g" "h" "i" "j" "k" "l" "m" "n" "o" "p" "q" "r" "s" "t" "u" "v" "w" "x" "y" "z" [[3]] [1] 25 26 27 28 29 30 31 32 y <- c(3,5,9)
I would like to extract from mylist the sub-elements 3,5, and 9 of each component in the list. I have tried,
sapply[mylist,"[[",y]
but not luck!, and others like vapply, lapply, etc.. Thanks in advance for your helpMauricio Ortiz
-
Paul Hiemstra about 12 yearsPlease use
lapply
, right now this solution does not scale at all whenmylist
becomes bigger. -
Swift Arrow over 4 yearsI have used this solution, but for some reason, it adds a column containing the index to each element. very confusing.