How to swap nibbles in C?
14,676
Solution 1
Start from the fact that hexadecimal 0xf
covers exactly four bits. There are four nibbles in a 16-bit number. The masks for the nibbles are 0xf000
, 0xf00
, 0xf0
, and 0xf
. Then start masking, shifting and bitwise OR-ing.
Solution 2
Sean Anderson's bit twiddling guide has the following:
// swap nibbles ...
v = ((v >> 4) & 0x0F0F0F0F) | ((v & 0x0F0F0F0F) << 4);
under the entry for Reverse an N-bit quantity in parallel in 5 * lg(N) operations.
Solution 3
1)
y = ((x >> 4) & 0x0f) | ((x << 4) & 0xf0);
2)
unsigned char swap_nibbles(unsigned char c)
{
unsigned char temp1, temp2;
temp1 = c & 0x0F;
temp2 = c & 0xF0;
temp1=temp1 << 4;
temp2=temp2 >> 4;
return(temp2|temp1); //adding the bits
}
3)
unsigned char nibbleSwap(unsigned char a)
{
return (a<<4) | (a>>4);
}
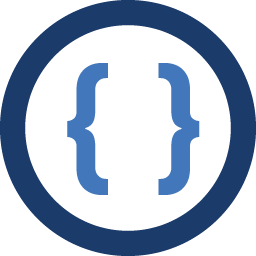
Author by
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
How to swap the nibble bit positions of a number?
For example: 534, convert it into binary, the rightmost 4 bits has to be interchanged with the leftmost 4 bits and then make a new number with that.
Anyone know how to do this?
-
Admin over 12 yearsActually, what is the difference between 0x0F and 0xF0 ?
-
Nikolai Fetissov over 12 years
0xf0
is 240 in decimal, while0x0f
is 15. -
Admin over 12 yearsI saw this example from one site..
unsigned char c= 0x34; temp1 = c & 0x0F; temp2 = c & 0xF0; temp1=temp1 << 4; temp2=temp2 >> 4; return(temp2|temp1);
What does that mean? -
Admin over 12 yearsI saw this example from one site..
unsigned char c= 0x34; temp1 = c & 0x0F; temp2 = c & 0xF0; temp1=temp1 << 4; temp2=temp2 >> 4; return(temp2|temp1);
What does that mean? -
JoeFish over 12 yearsIt means: assign the value 0x34 to c; store the bottom 4 bits of c in temp1; store the top 4 bits of c in temp2; shift the bits in temp1 4 places left; shift the bits in temp2 4 places right; OR temp2 and temp1 together to create an 8-bit value. Do some Google searching for bit manipulation and it will make more sense.
-
sarnold over 12 yearsNote that this is for reconstructing a 32 bit integer from an array of bytes. It makes an assumption about the endianness of the host processor and thus cannot be blindly used in programs -- there must be a corresponding
decode_32le()
routine that works on platforms of the opposite endianness in case this code is compiled on such a platform. It's also unrelated to nibble swapping, since it arranges bytes. -
subbupkb over 12 yearsyes, your very right. hence forth i had mentioned its for 32 bit Endiness swap. thanks for more clarification.
-
LtWorf over 2 yearsYou forgot to make sure the bitmask size is correct with sizeof, remembering that there are no size guarantees in C
-
ramrunner over 2 yearswould you mind fixing the code a bit with consistent indentation and having main actually return something? the code displayed in the site should be high quality and pass a code review cleanly if possible.