how to take jave heap dump every 30 seconds using kill -3 <pid> command
17,563
Solution 1
Have you tried such a simple shell script?
while true
do
jmap -dump:file=/tmp/java-`date +%s`.hprof PID_OF_JVM
sleep 30
done
This will create one file pear each snapshot. For thread dump you can use similar script:
while true
do
jstack PID_OF_JVM > stack-`date +%s`.txt
sleep 30
done
I guess you can use kill -3
instead of jstack
.
Solution 2
you can do thread dumping from java application using code like this
public static String getDumpFor(Thread thread) {
StringBuilder sb = new StringBuilder();
if (thread.isAlive()) {
StackTraceElement[] stackTrace = thread.getStackTrace();
sb.append(thread.toString()).append("\n")
.append(String.format(" State - %s,", thread.getState()))
.append(String.format(" Is daemon = %s,", thread.isDaemon()));
for (StackTraceElement s : stackTrace)
sb.append("\tat ").append(s.getClassName()).append(".").append(s.getMethodName()).append("(").append(s.getFileName()).append(":").append(s.getLineNumber()).append(")")
.append("\n");
}
return sb.toString();
}
public static void dumpActiveThreads() {
Map<Thread, StackTraceElement[]> stackTraces = Thread.getAllStackTraces();
Set<Thread> keySet = stackTraces.keySet();
System.out.println("\nThread dump begin:");
for (Thread thread : keySet)
dumpActiveThread(thread);
System.out.println("\nThread dump end.");
}
and then schedule task like this
final ScheduledFuture<?> scheduledFuture = scheduledExecutorService.scheduleWithFixedDelay(
new Runnable() {dumpActiveThreads()},
0,
30, TimeUnit.SECONDS);
Solution 3
I have not used kill -3
command but i have used jmap
command provided by sun sdk
You can write a script and then in script run below command.
${JAVA_HOME}/bin/jmap -dump:file=/home/MyDump.hprof PID
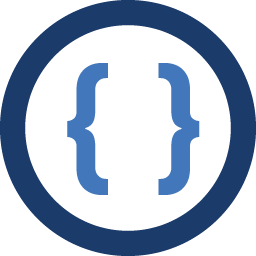
Author by
Admin
Updated on June 28, 2022Comments
-
Admin almost 2 years
Please help in this, i want to run a shell script where it should take jave heap dump every 30 seconds using kill -3 command. Thanks in advance.