How to trigger an event in input text after I stop typing/writing?
Solution 1
You'll have to use a setTimeout
(like you are) but also store the reference so you can keep resetting the limit. Something like:
//
// $('#element').donetyping(callback[, timeout=1000])
// Fires callback when a user has finished typing. This is determined by the time elapsed
// since the last keystroke and timeout parameter or the blur event--whichever comes first.
// @callback: function to be called when even triggers
// @timeout: (default=1000) timeout, in ms, to to wait before triggering event if not
// caused by blur.
// Requires jQuery 1.7+
//
;(function($){
$.fn.extend({
donetyping: function(callback,timeout){
timeout = timeout || 1e3; // 1 second default timeout
var timeoutReference,
doneTyping = function(el){
if (!timeoutReference) return;
timeoutReference = null;
callback.call(el);
};
return this.each(function(i,el){
var $el = $(el);
// Chrome Fix (Use keyup over keypress to detect backspace)
// thank you @palerdot
$el.is(':input') && $el.on('keyup keypress paste',function(e){
// This catches the backspace button in chrome, but also prevents
// the event from triggering too preemptively. Without this line,
// using tab/shift+tab will make the focused element fire the callback.
if (e.type=='keyup' && e.keyCode!=8) return;
// Check if timeout has been set. If it has, "reset" the clock and
// start over again.
if (timeoutReference) clearTimeout(timeoutReference);
timeoutReference = setTimeout(function(){
// if we made it here, our timeout has elapsed. Fire the
// callback
doneTyping(el);
}, timeout);
}).on('blur',function(){
// If we can, fire the event since we're leaving the field
doneTyping(el);
});
});
}
});
})(jQuery);
$('#example').donetyping(function(){
$('#example-output').text('Event last fired @ ' + (new Date().toUTCString()));
});
<script src="https://ajax.googleapis.com/ajax/libs/jquery/1.11.1/jquery.min.js"></script>
<input type="text" id="example" />
<p id="example-output">Nothing yet</p>
That will execute when:
- The timeout has elapsed, or
- The user switched fields (
blur
event)
(Whichever comes first)
Solution 2
SOLUTION:
Here is the solution. Executing a function after the user has stopped typing for a specified amount of time:
var delay = (function(){
var timer = 0;
return function(callback, ms){
clearTimeout (timer);
timer = setTimeout(callback, ms);
};
})();
Usage
$('input').keyup(function() {
delay(function(){
alert('Hi, func called');
}, 1000 );
});
Solution 3
You can use underscore.js "debounce"
$('input#username').keypress( _.debounce( function(){<your ajax call here>}, 500 ) );
This means that your function call will execute after 500ms of pressing a key. But if you press another key (another keypress event is fired) before the 500ms, the previous function execution will be ignored (debounced) and the new one will execute after a fresh 500ms timer.
For extra info, using _.debounce(func,timer,true) would mean that the first function will execute and all other keypress events withing subsequent 500ms timers would be ignored.
Solution 4
You need debounce!
Here is a jQuery plugin, and here is all you need to know about debounce. If you are coming here from Google and Underscore has found its way into the JSoup of your app, it has debounce baked right in!
Solution 5
You should assign setTimeout
to a variable and use clearTimeout
to clear it on keypress.
var timer = '';
$('input#username').keypress(function() {
clearTimeout(timer);
timer = setTimeout(function() {
//Your code here
}, 3000); //Waits for 3 seconds after last keypress to execute the above lines of code
});
Hope this helps.
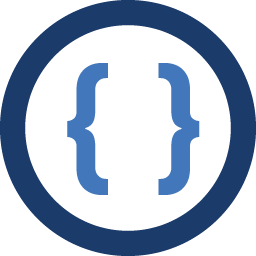
Admin
Updated on August 05, 2021Comments
-
Admin over 2 years
I want to trigger an event just after I stop typing (not while typing) characters in my input textbox.
I've tried with:
$('input#username').keypress(function() { var _this = $(this); // copy of this object for further usage setTimeout(function() { $.post('/ajax/fetch', { type: 'username', value: _this.val() }, function(data) { if(!data.success) { // continue working } else { // throw an error } }, 'json'); }, 3000); });
But this example produces a timeout for every typed character and I get about 20 AJAX requests if I type-in 20 characters.
On this fiddle I demonstrate the same problem with a simple alert instead of an AJAX.
Is there a solution for this or I'm just using a bad approach for this?