How to turn all numbers in a list into their negative counterparts?
53,660
Solution 1
The most natural way is to use a list comprehension:
mylist = [ 1, 2, 3, -7]
myneglist = [ -x for x in mylist]
print(myneglist)
Gives
[-1, -2, -3, 7]
Solution 2
If you want to modify a list in place:
mylist = [ 1, 2, 3, -7]
print(mylist)
for i in range(len(mylist)):
mylist[i] = -mylist[i]
print(mylist)
Solution 3
You can use the numpy package and do numpy.negative()
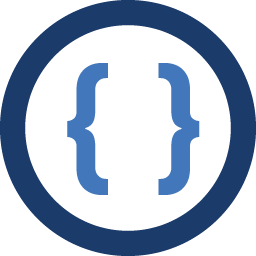
Author by
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
I am trying to turn a list of positive numbers into a list of negative numbers with the same value in python 3.3.3
For example turning
[1,2,3]
into[-1,-2,-3]
I have this code:
xamount=int(input("How much of x is there")) integeramount=int(input("How much of the integer is there")) a=1 lista=[] while(a<=integeramount): if(integeramount%a==0): lista.extend([a]) a=a+1 listb=lista print(listb) [ -x for x in listb] print(listb)
This prints two identical lists when I want one to be positive and one to be negative.
-
Admin over 9 yearsUnfortunately this works when typed into shell, however when put into code it does not work, is there an alternative?
-
Anton over 9 yearsYou are mistaken. Everything that works in the shell works "in code". If you have something that doesn't work and need help, you need to post code that shows what you are trying to do.
-
pepr over 9 years@Anton: Do not put the
>>>
to the code. Otherwise, it must work also with possibly the oldest Python you can find. The only problem could be that it creates the new list. It does not modify the existing one. -
Admin over 9 yearsThanks @pepr that was the problem
-
Anton over 9 years@pepr, OK, I removed the
>>>
. -
pepr over 9 years@Anton: Sorry. The note was meant to SamRob85 :) It is OK to use the interactive Python prefix as even the beginners understand that it is not part of the code written into
.py
file. It just flashed to me that it could be the problem in the SamRob's case.